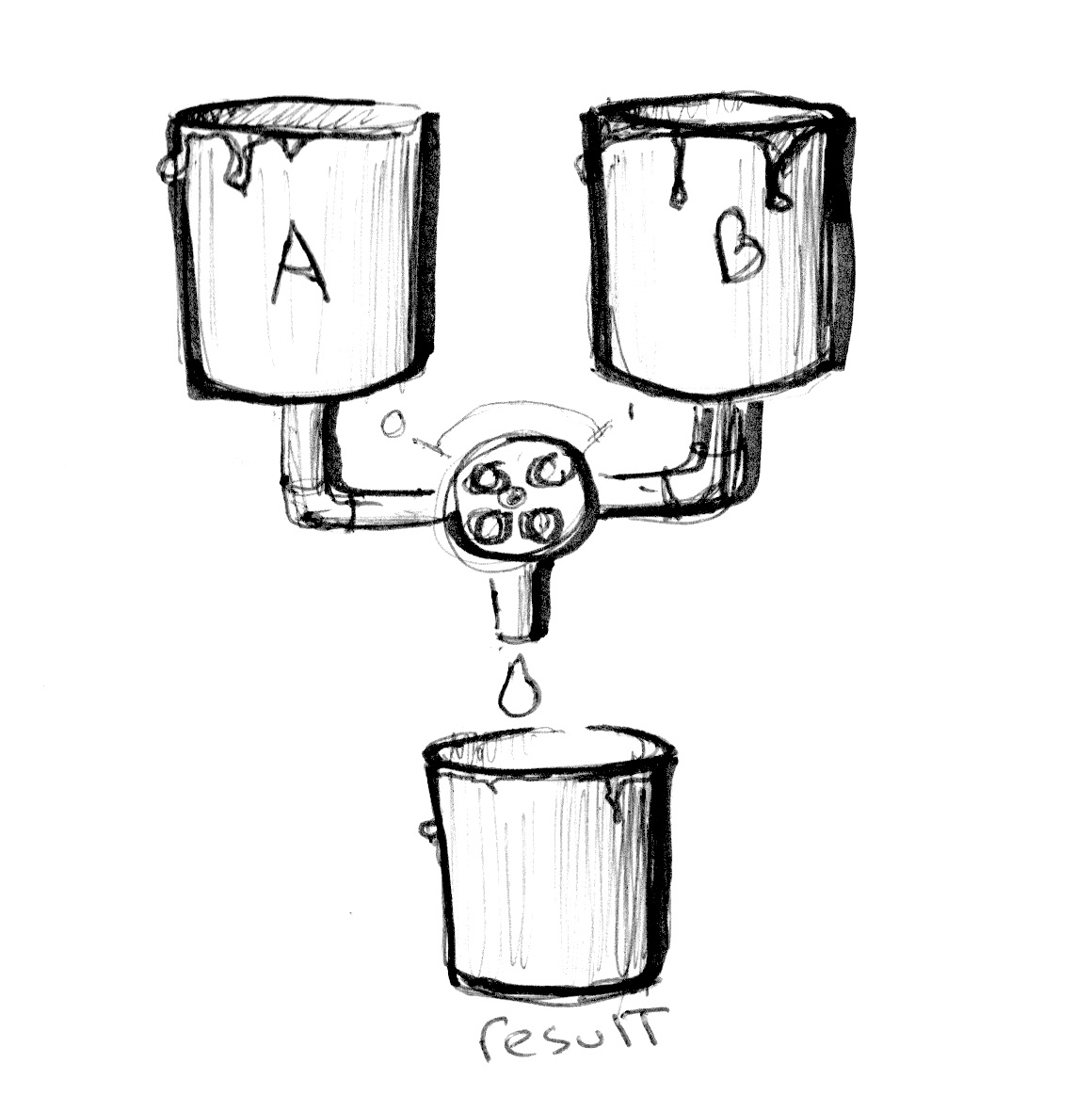
Check the following code at line 18 and see how we are using the absolute values of a sin wave over time to mix colorA and colorB.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform float u_time;
vec3 colorA = vec3(0.149,0.141,0.912);
vec3 colorB = vec3(1.000,0.833,0.224);
void main() {
vec3 color = vec3(0.0);
float pct = abs(sin(u_time));
// Mix uses pct (a value from 0-1) to
// mix the two colors
color = mix(colorA, colorB, pct);
gl_FragColor = vec4(color,1.0);
}
The function mix() mixs colorA and colorB with the percantage, pct. If the pct is 0, it print colorA. Like this:
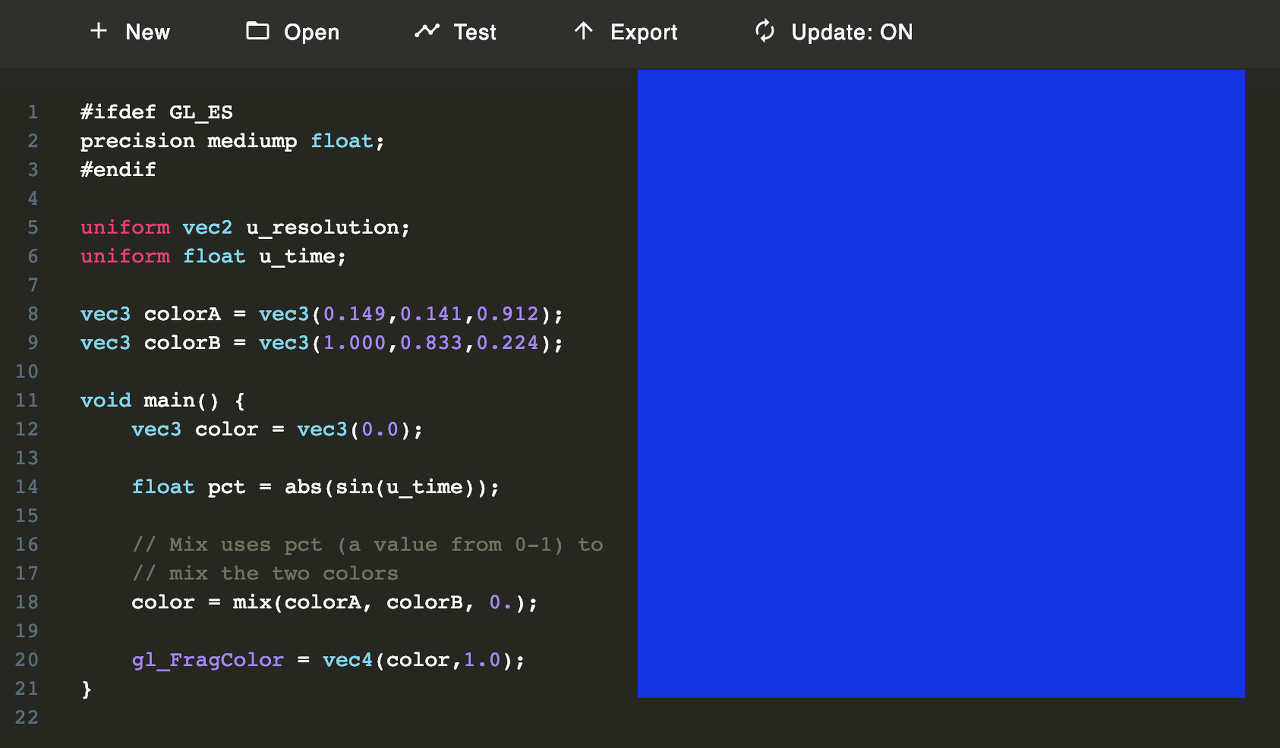
If the pct is 1, it print colorB like this:
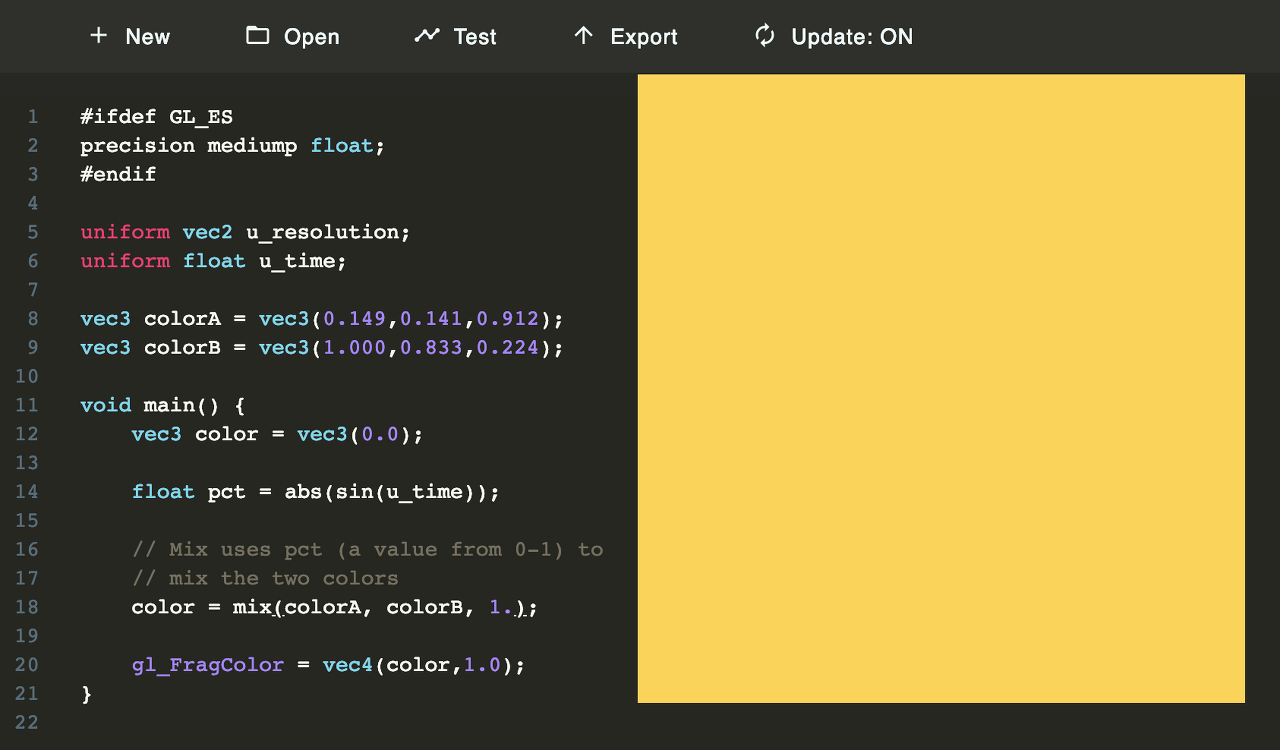
The mix( ) function has more to offer. Instead of a single float, we can pass a variable type that matches the two first arguments, in our case a vec3. By doing that we gain control over the mixing percentages of each individual color channel, r, g and b.
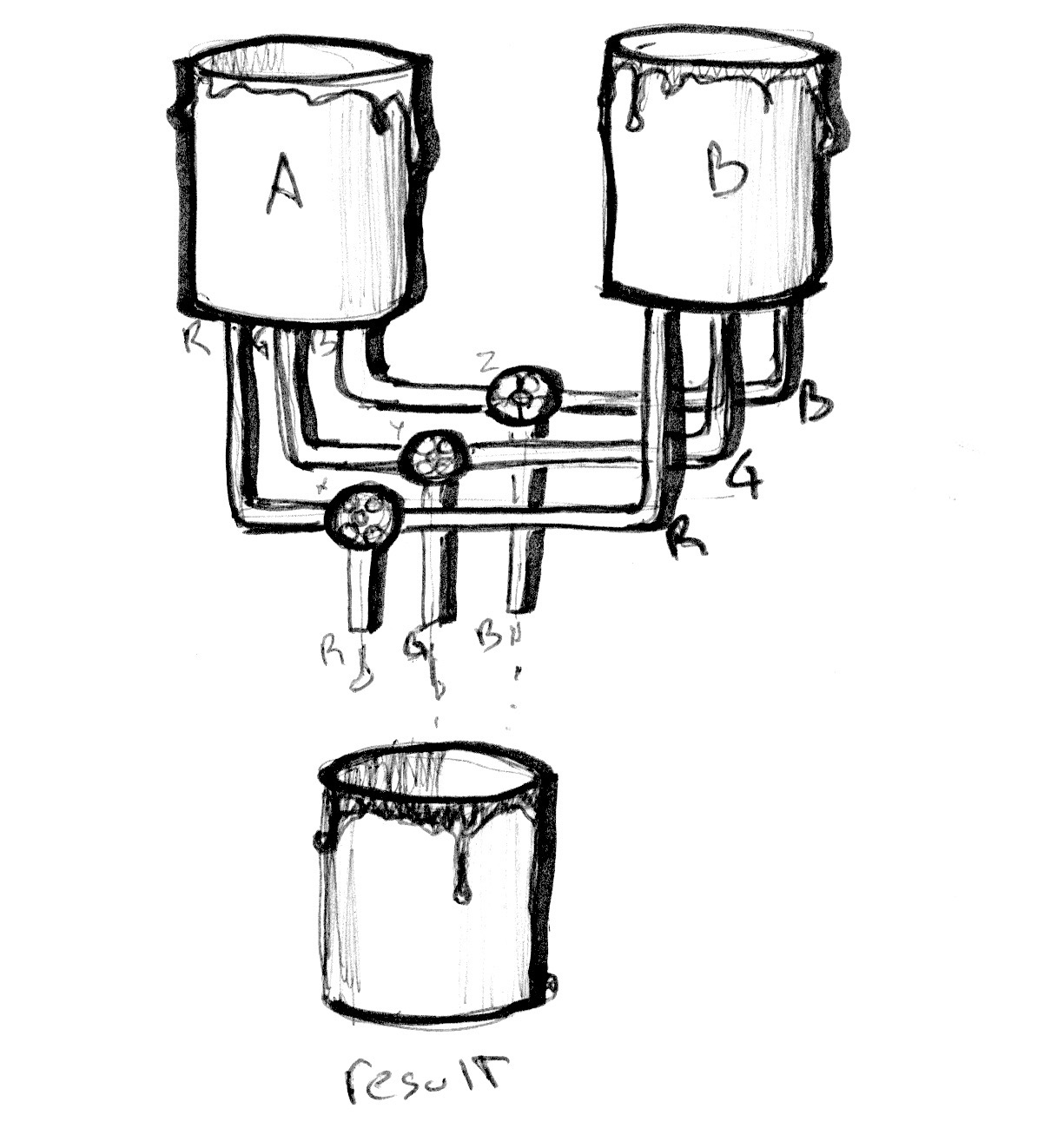
#ifdef GL_ES
precision mediump float;
#endif
#define PI 3.14159265359
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 colorA = vec3(0.149,0.141,0.912);
vec3 colorB = vec3(1.000,0.833,0.224);
float plot (vec2 st, float pct){
return smoothstep( pct-0.01, pct, st.y) -
smoothstep( pct, pct+0.01, st.y);
}
void main() {
vec2 st = gl_FragCoord.xy/u_resolution.xy;
vec3 color = vec3(0.0);
vec3 pct = vec3(st.x);
// pct.r = smoothstep(0.0,1.0, st.x);
// pct.g = sin(st.x*PI);
// pct.b = pow(st.x,0.5);
color = mix(colorA, colorB, pct);
// Plot transition lines for each channel
color = mix(color,vec3(1.0,0.0,0.0),plot(st,pct.r));
color = mix(color,vec3(0.0,1.0,0.0),plot(st,pct.g));
color = mix(color,vec3(0.0,0.0,1.0),plot(st,pct.b));
gl_FragColor = vec4(color,1.0);
}
Now I can control the mixing percentage by allocating different values at pct.r, pct.g, and pct.b.
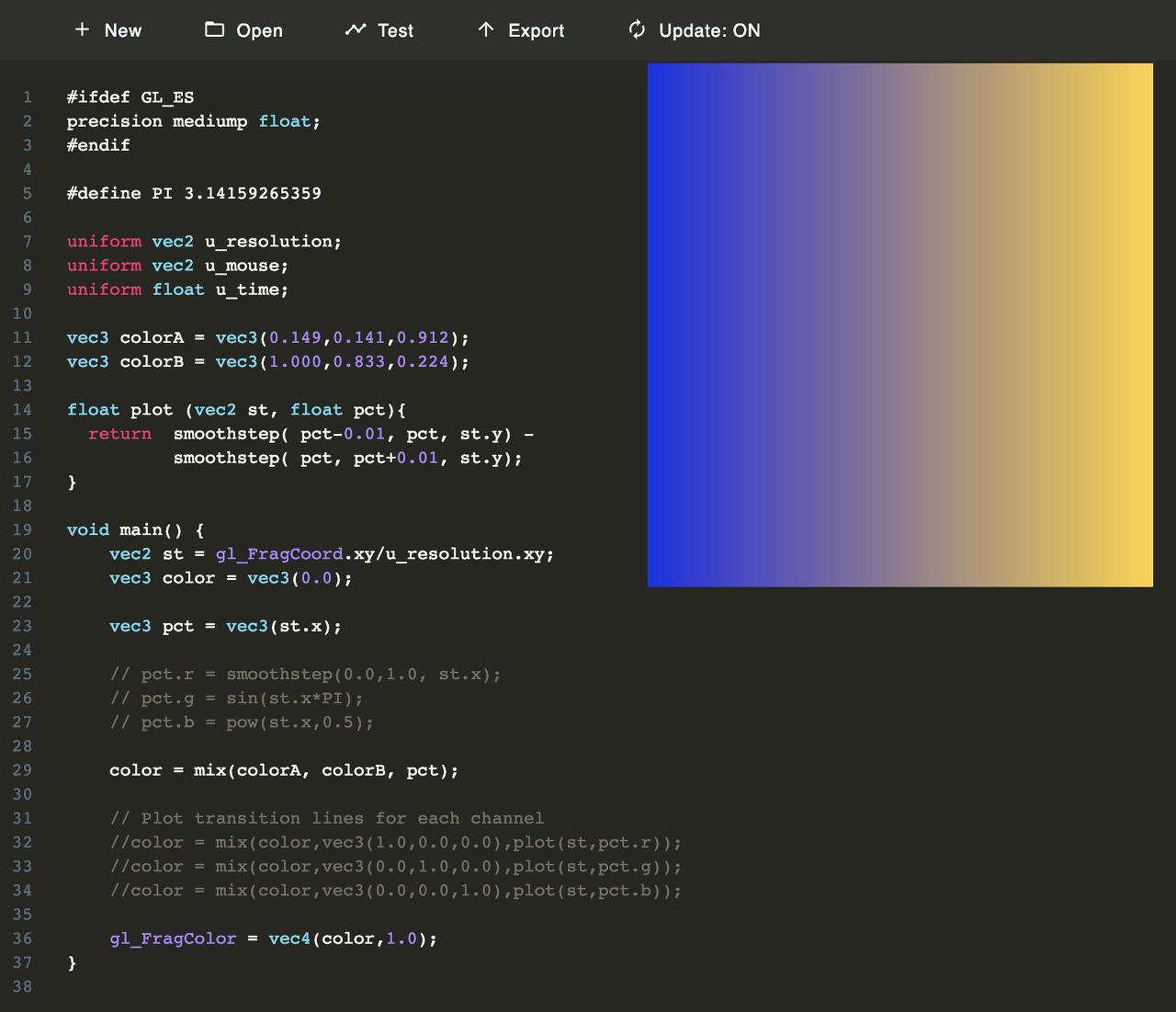
control red with smoothstep
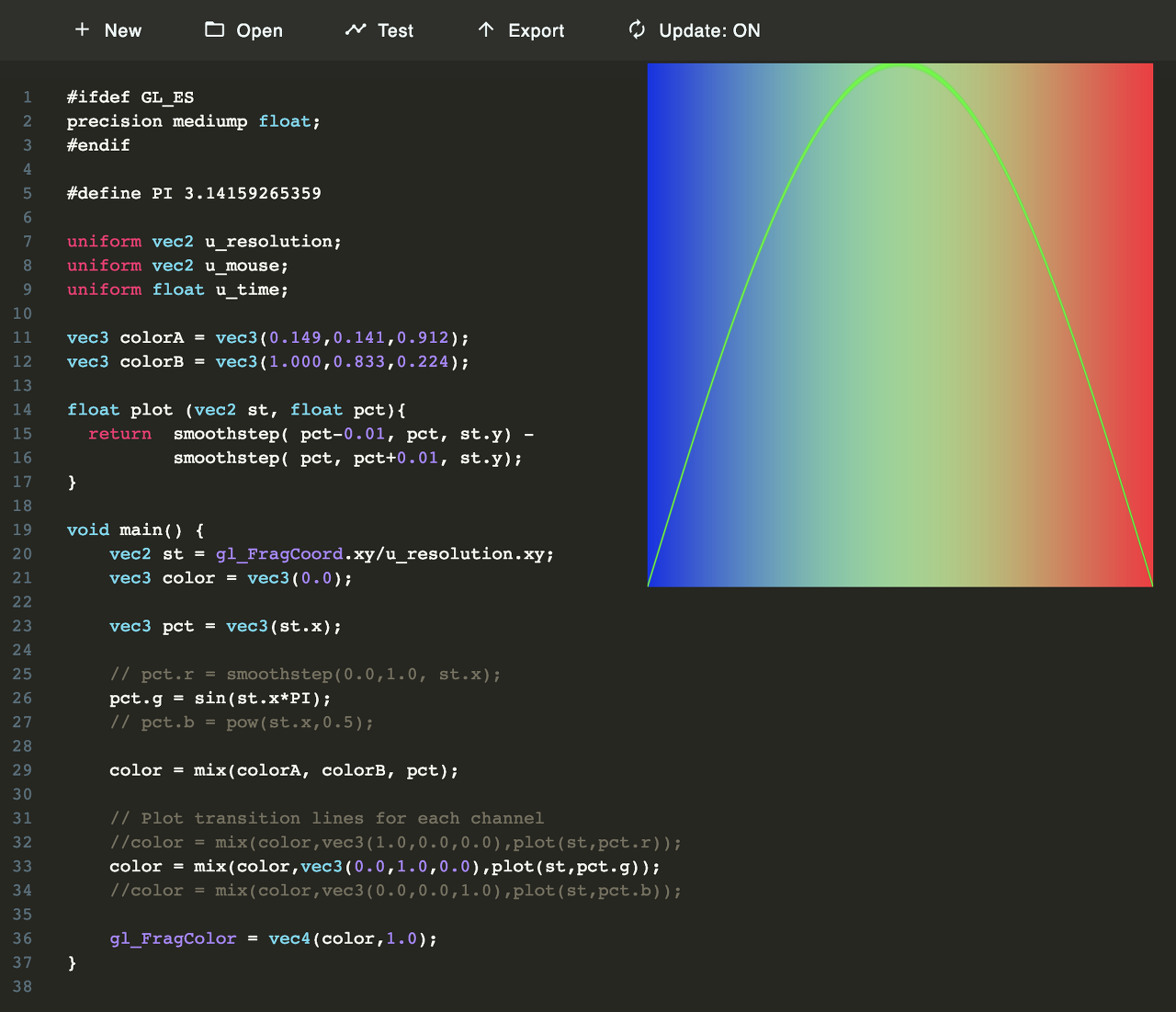
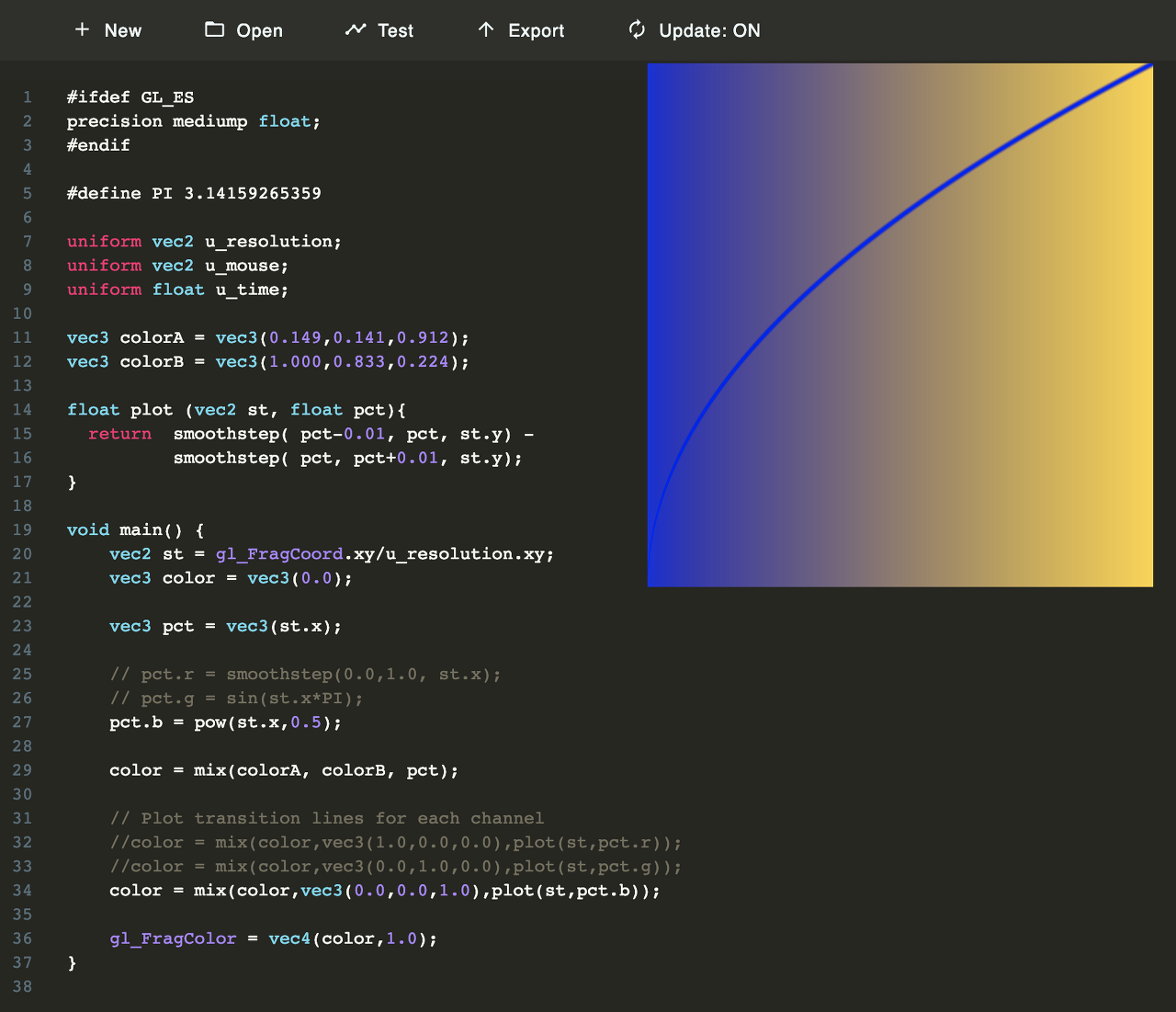
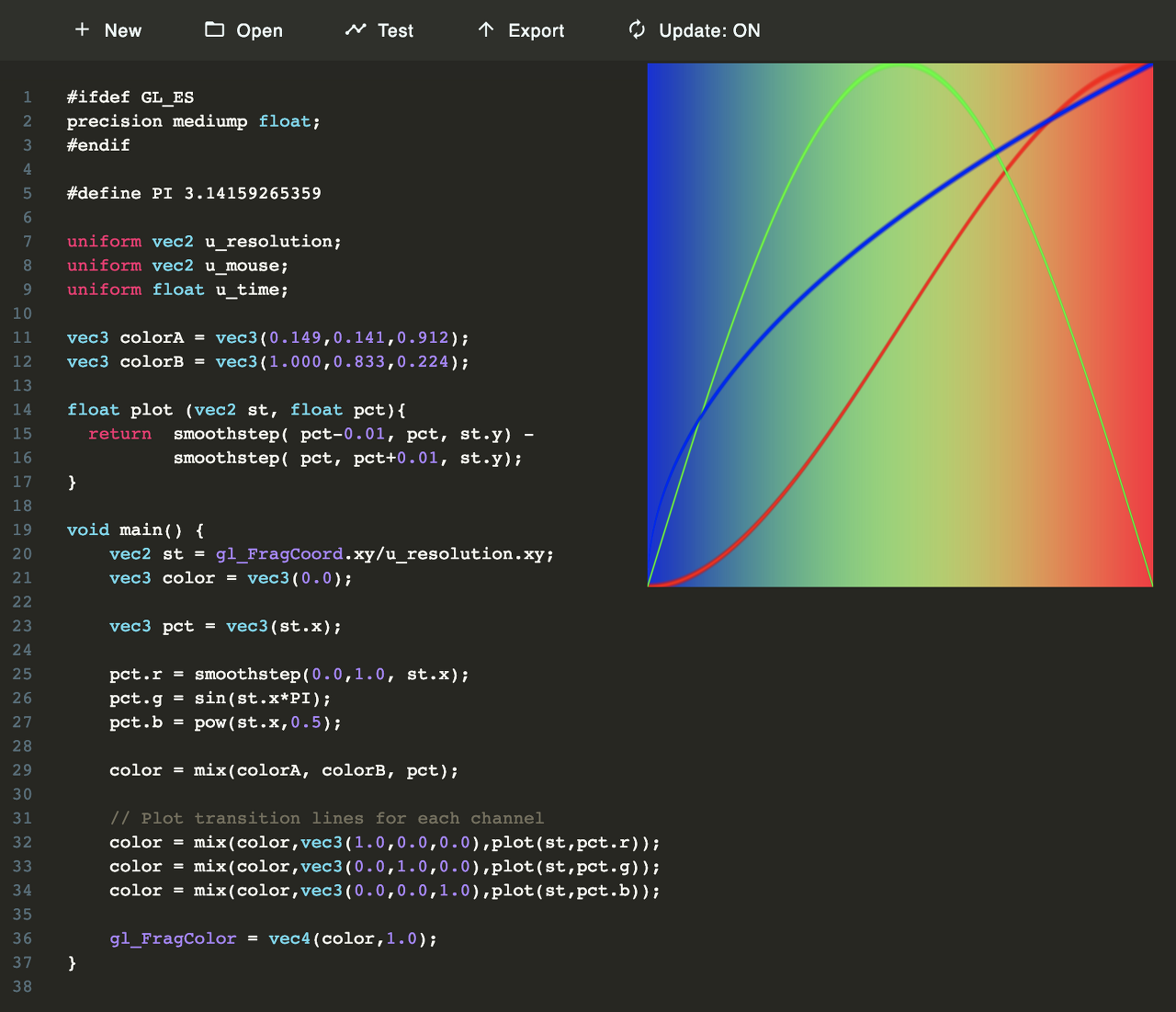
The percent control above mixes r, g, and b, respectively. The visualized line graph shows the percentage value (y = pct) at each x-coordinate. At that point, the mix function overwrites the r, g, and b values of colorA (blue) with the r, g, and b values of colorB (yellow) at the rate of pct.
*헷갈려서 써놓는 한글 설명: 위의 퍼센트 컨트롤은 r, g, b를 각각 섞을 수 있도록 도와준다. 비주얼화된 선 그래프는 각각의 x좌표에서의 퍼센트(y = pct) 값이 얼마인지를 나타낸다. 해당 지점에서 mix 함수는 colorA(파란색)의 r, g, b 값에 colorB(노란색)의 r, g, b 값을 pct의 값 만큼의 비율로 덮어 출력한다.
Can you make a rainbow using what we have learned so far?
- Yeah. It's a little bit weird but not bad...
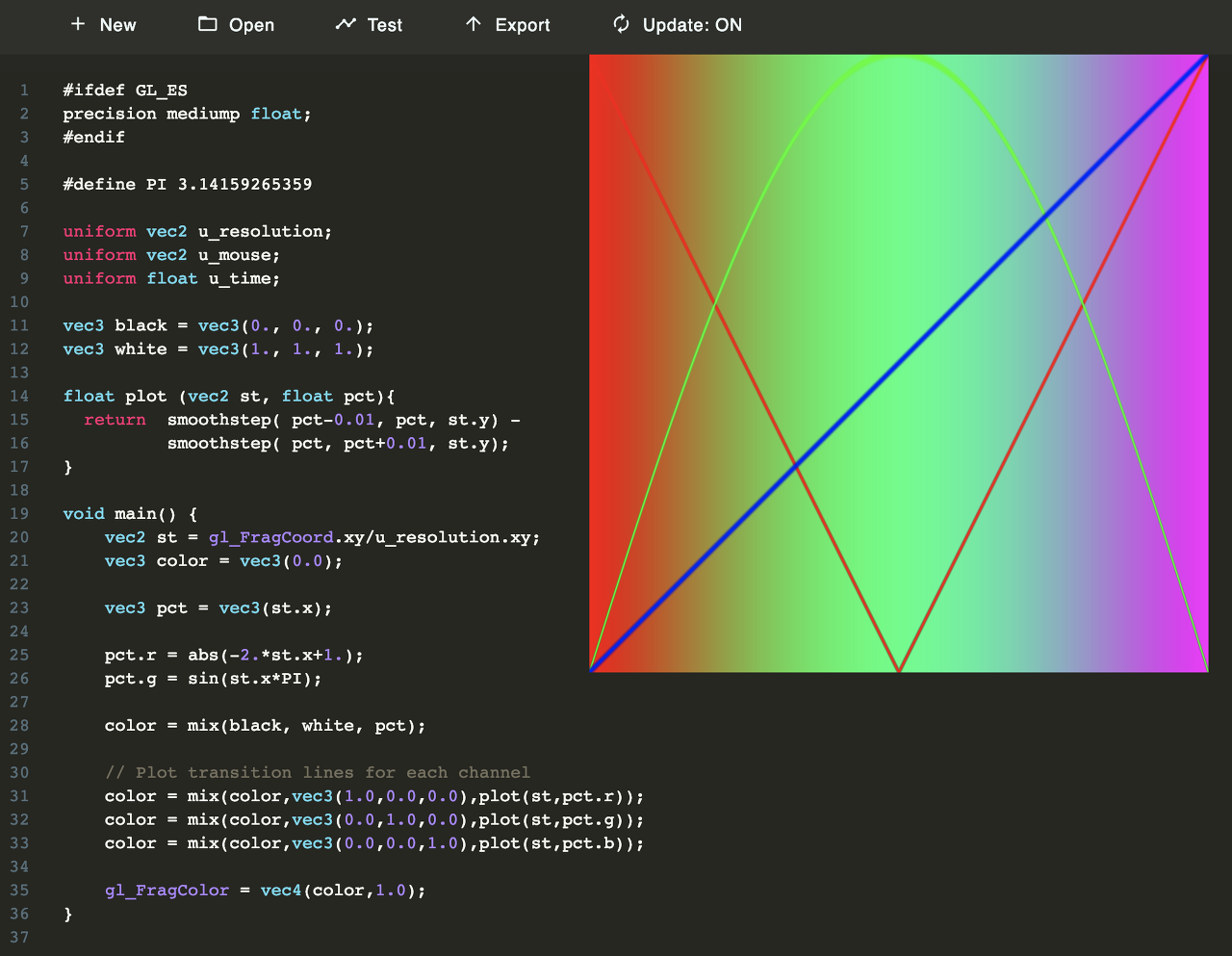
#ifdef GL_ES
precision mediump float;
#endif
#define PI 3.14159265359
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 black = vec3(0., 0., 0.);
vec3 white = vec3(1., 1., 1.);
float plot (vec2 st, float pct){
return smoothstep( pct-0.01, pct, st.y) -
smoothstep( pct, pct+0.01, st.y);
}
void main() {
vec2 st = gl_FragCoord.xy/u_resolution.xy;
vec3 color = vec3(0.0);
vec3 pct = vec3(st.x);
pct.r = abs(-2.*st.x+1.);
pct.g = sin(st.x*PI);
color = mix(black, white, pct);
// Plot transition lines for each channel
color = mix(color,vec3(1.0,0.0,0.0),plot(st,pct.r));
color = mix(color,vec3(0.0,1.0,0.0),plot(st,pct.g));
color = mix(color,vec3(0.0,0.0,1.0),plot(st,pct.b));
gl_FragColor = vec4(color,1.0);
}
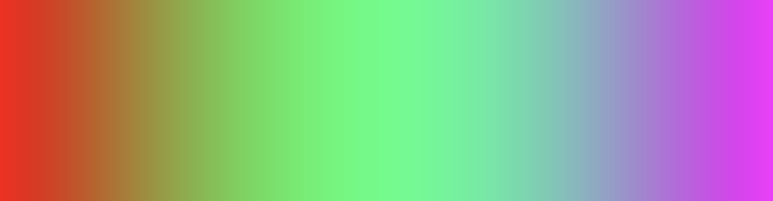
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Qualifier (0) | 2022.01.05 |
---|---|
[GLSL] Atan (0) | 2022.01.05 |
[GLSL] Color (0) | 2022.01.05 |
[GLSL] Gain (0) | 2022.01.05 |
[GLSL] Shaping Functions (0) | 2022.01.05 |
댓글