#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main() {
vec2 st = gl_FragCoord.xy/u_resolution;
float y = st.x;
vec3 color = vec3(y);
gl_FragColor = vec4(color,1.0);
}
With the above code, we can make the gradient from black to white.
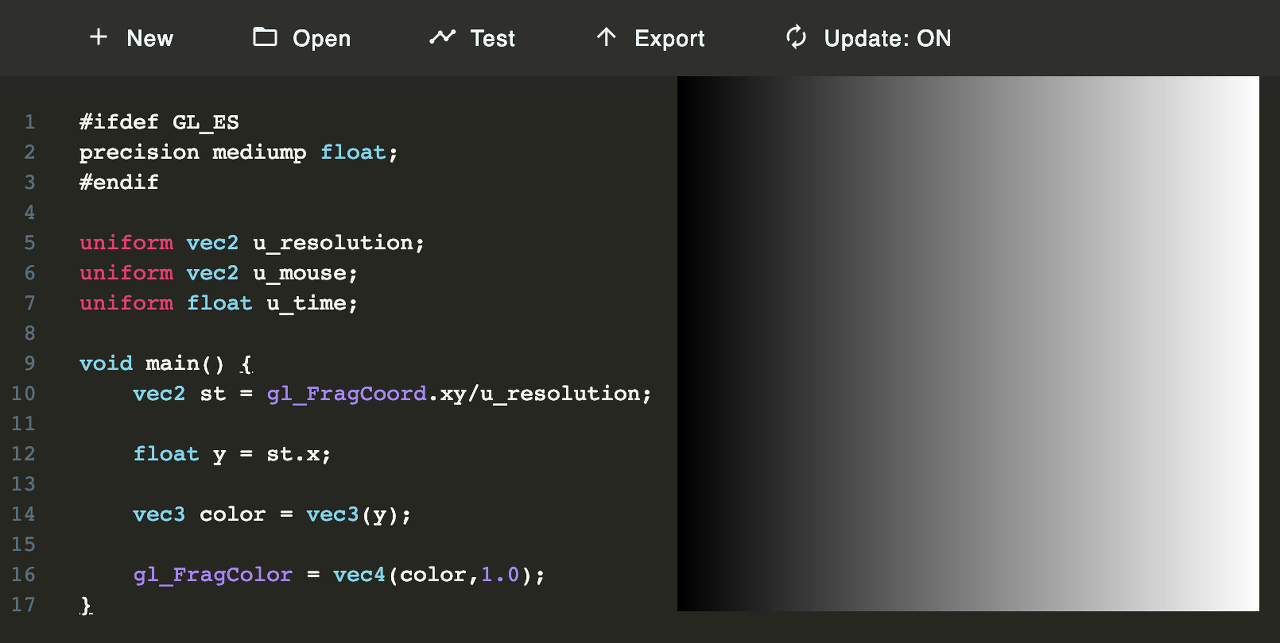
If the type 'vec3' has only one parameter, it assigns the same values with the one. So in line 14, it is same as 'vec3(y,y,y)'. Oh, the book mentions it too.
The vec3 type constructor "understands" that you want to assign the three color channels with the same value, while vec4 understands that you want to construct a four dimensional vector with a three dimensional one plus a fourth value (in this case the value that controls the alpha or opacity).
Now let's look at a deeper code.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
// Plot a line on Y using a value between 0.0-1.0
float plot(vec2 st, float pct){
return smoothstep( pct-0.02, pct, st.y) -
smoothstep( pct, pct+0.02, st.y);
}
void main() {
vec2 st = gl_FragCoord.xy/u_resolution;
float y = st.x;
vec3 color = vec3(y);
// Plot a line
float pct = plot(st,y);
color = (1.0-pct)*color+pct*vec3(0.0,1.0,0.0);
gl_FragColor = vec4(color,1.0);
}
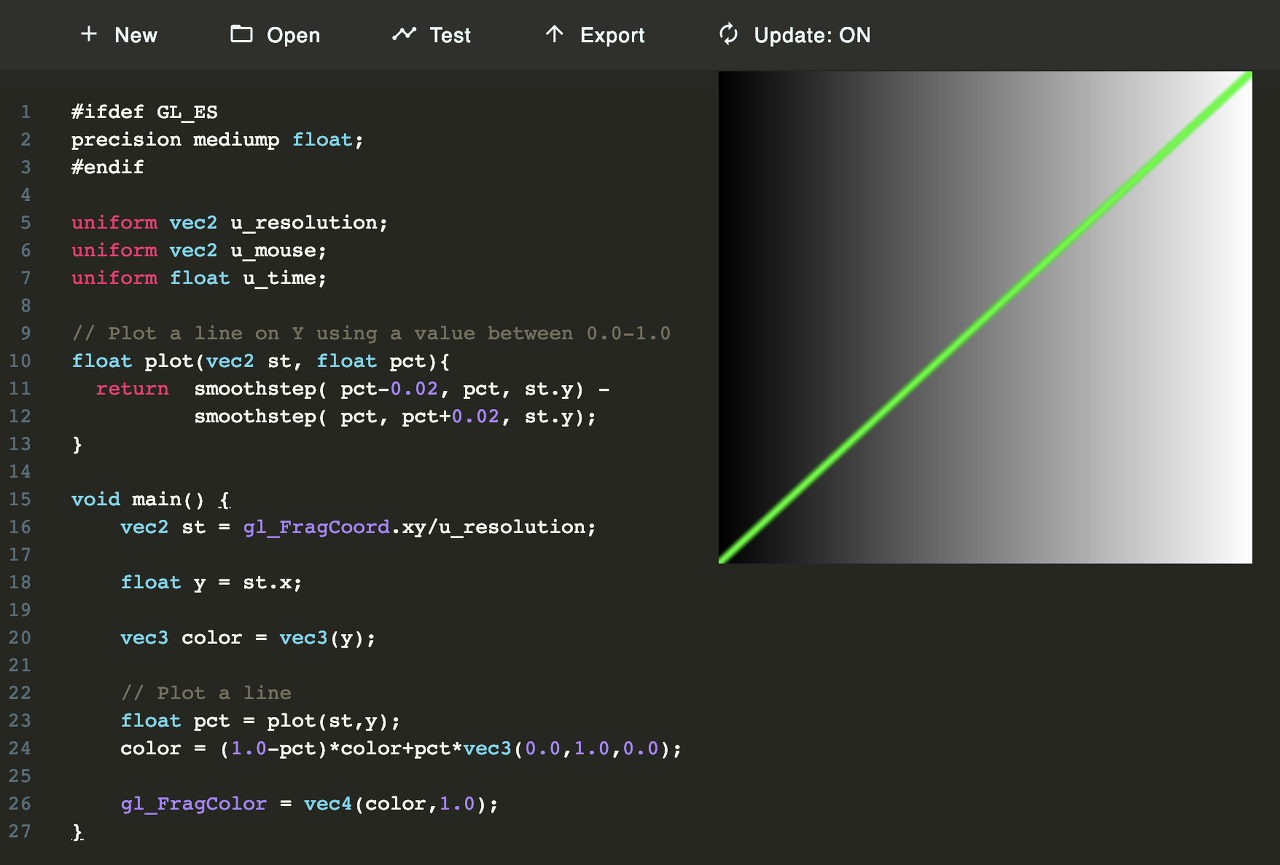
In it, we visualize the normalized value of the x coordinate (st.x) in two ways: one with brightness (observe the nice gradient from black to white) and the other by plotting a green line on top (in that case the x value is assigned directly to y).
Let's search the function 'smoothstep'.
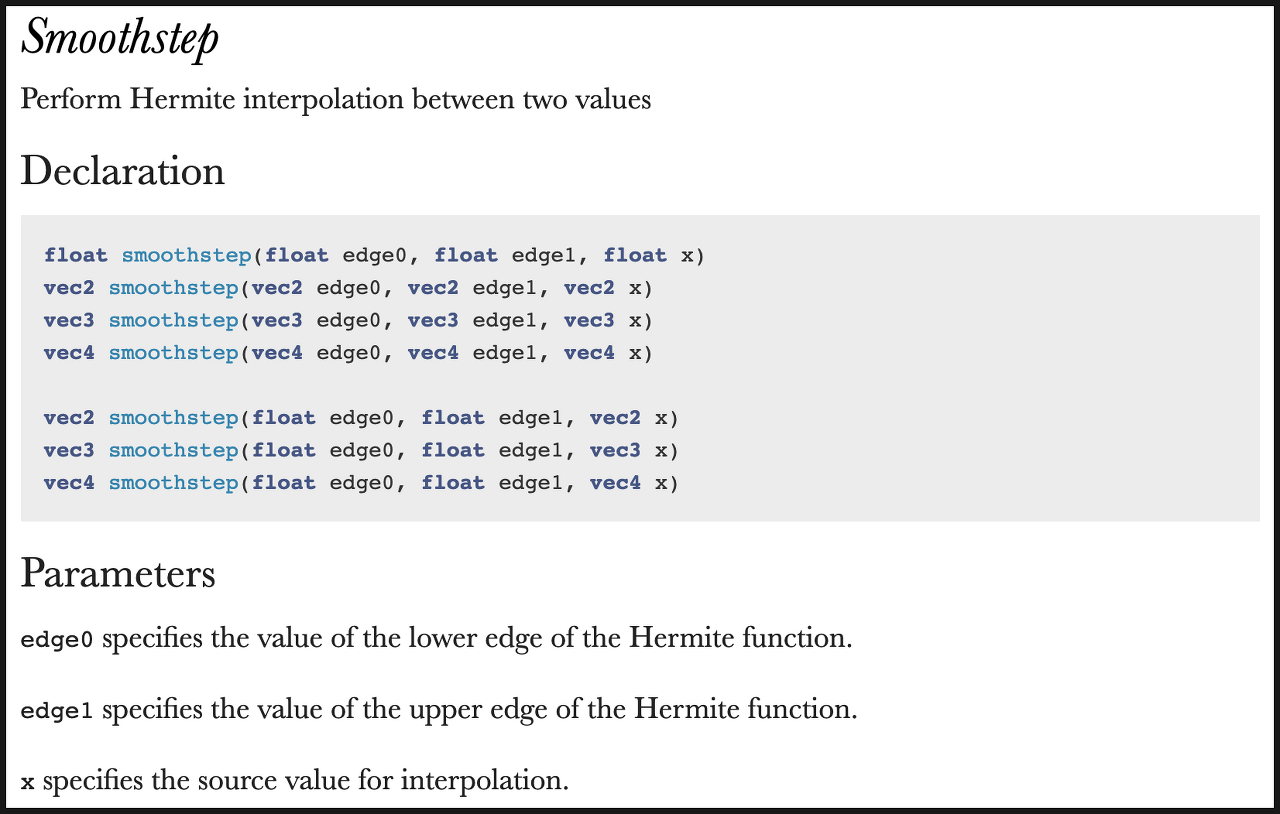
As you can see, it can be used in many declarations. But, usually the first definition is used. It means:
- case 1. If the x value is less than edge0, it returns 0.
- case 2. If the x value is greater than edge1, it returns 1.
- case 3. If the x value is between edge0 and 1, it returns the value between 0 and 1 (which gentle increase.)
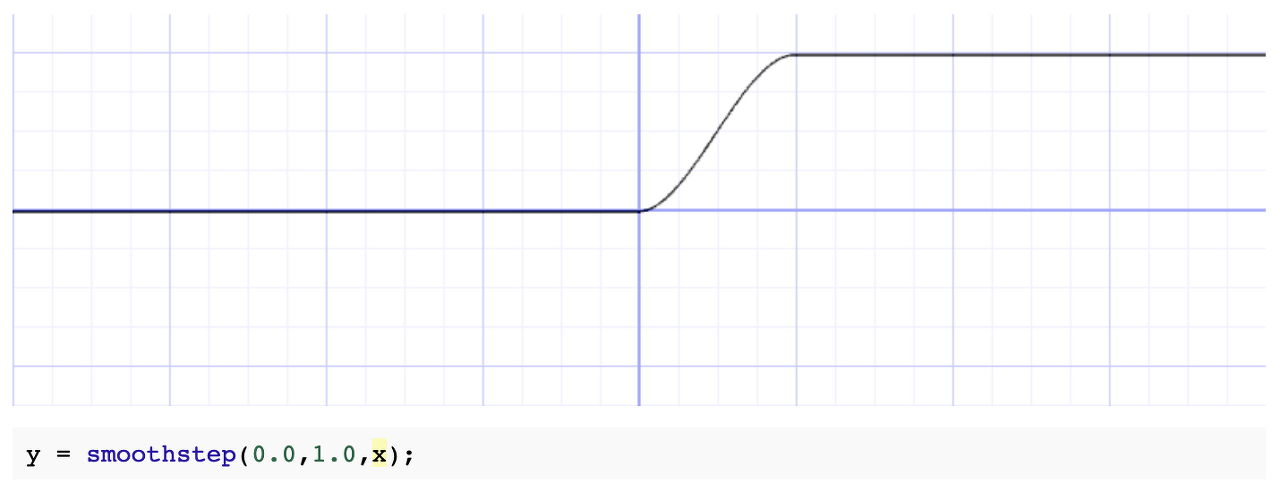
So, let's go back to the codes. In line 23, the function 'plot' is called, and it gets the arguments st and y(st.x). So the function 'plot' returns 'smoothstep( st.x-0.02, st.x, st.y) - smoothstep( st, st.x+0.02, st.y)'.
Let's divide a region of pixels with the same x-coordinate. If the y-coordinate of the pixel (normalized between 0 and 1) is less then 'x-coordinate(also normalized) - 0.02', it belongs to region 1. If the y-coord is between 'x-coord - 0.02' and 'x-coord', it belongs to region 2. If the y-coord is between 'x-coord' and 'x-coord + 0.02', it belongs to region 3. And last, if the y-coord is greater than 'x-coord + 0.02', it belongs to region 4.
If the pixel belongs region 1, the function 'plot' returns 0 - 0, 0. If belongs region 2, returns 0~1 - 0, 0~1. If belongs region 3, returns 0~1 - 1, 1~0. And if belongs region 4, returns 1 - 1, 0.
So, the return values are 0, 0~1, 1~0, 0. Then in line 24, the color don't be replaced when pct is 0, or not, it is mixed with green color in level of pct. (It is same as the built-in fuction mix().)
If I replace the value '0.02' with a variable 'span' and change its value, it will looks like:
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
// Plot a line on Y using a value between 0.0-1.0
float plot(vec2 st, float pct){
float span = 0.02;
return smoothstep( pct-span, pct, st.y) -
smoothstep( pct, pct+span, st.y);
}
void main() {
vec2 st = gl_FragCoord.xy/u_resolution;
float y = st.x;
vec3 color = vec3(y);
// Plot a line
float pct = plot(st,y);
color = (1.0-pct)*color+pct*vec3(0.0,1.0,0.0);
gl_FragColor = vec4(color,1.0);
}
Next time, let's look at various shaping functions including smoothstep.
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Gain (0) | 2022.01.05 |
---|---|
[GLSL] Shaping Functions (0) | 2022.01.05 |
[GLSL] Fragment Coordinate (gl_FragCoord) (0) | 2022.01.05 |
[GLSL] Uniform (0) | 2022.01.05 |
[GLSL] Hello World (0) | 2022.01.05 |
댓글