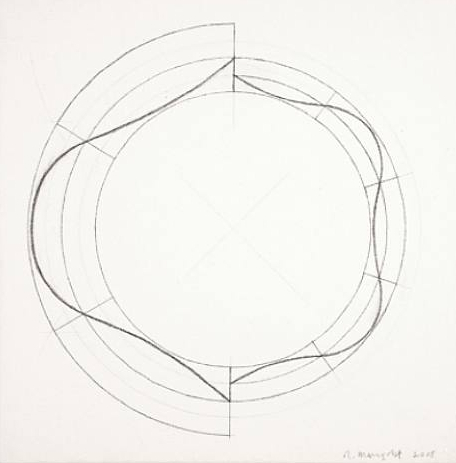
A circle is a figure with the same radius regardless of the angle from the center. But this time, let's make a shape that changes the distance to the center according to the angle.
Declare the variables a, d, and r in the default code.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
float a;
float d;
vec3 col;
gl_FragColor = vec4(col, 1.0);
}
'a' for the angle(0~PI, 0~-PI), 'd' for the distance of pixel to (0,0), and 'r' for radius(Actually, it is not a radius.).
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
float a = atan(coord.y, coord.x);
float d = length(coord);
vec3 col;
gl_FragColor = vec4(col, 1.0);
}
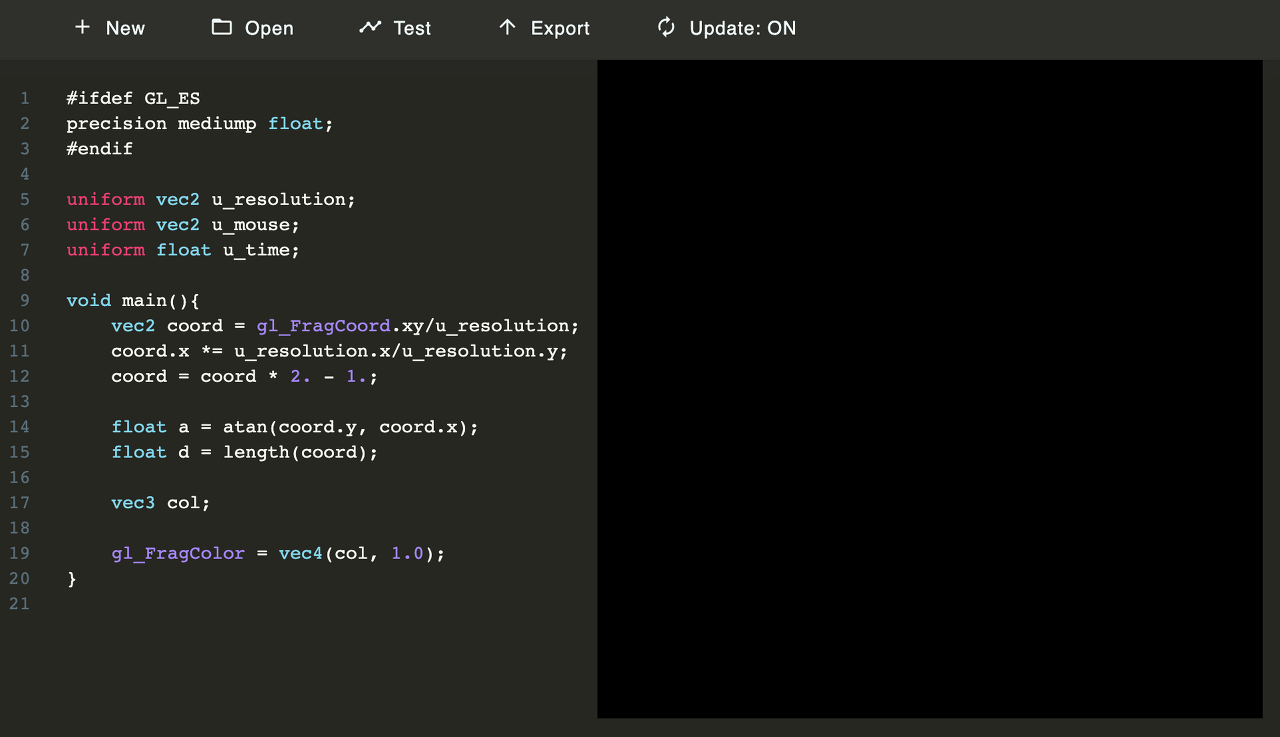
Let's set 'r' sin(a), then as the angle increases, the radius changes according to the sine function period. If 'a' is multiplied by an integer, that number of cycles from 0 to 1, 1 to 0 is repeated.
In the graph below, it can be seen that the sin (x) value is negative from -PI to 0, and when the value of x increases from 0 to PI, the y value increases from 0 to 1 and decreases again. In this area, the distance from the origin to the figure increases from 0 to 1 and decreases again.
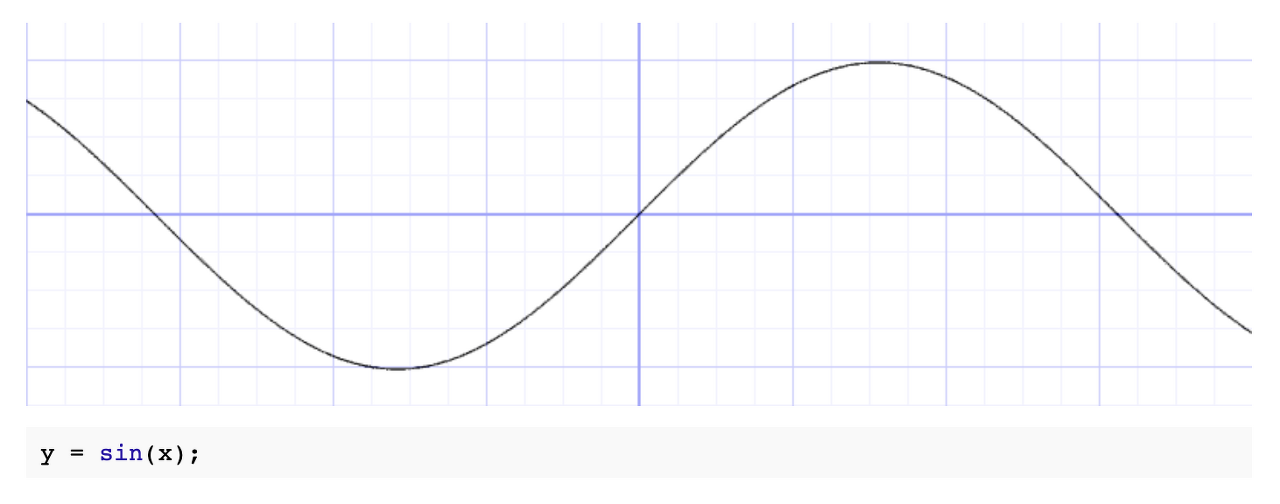
When 'r' is negative, the length can not be displayed.
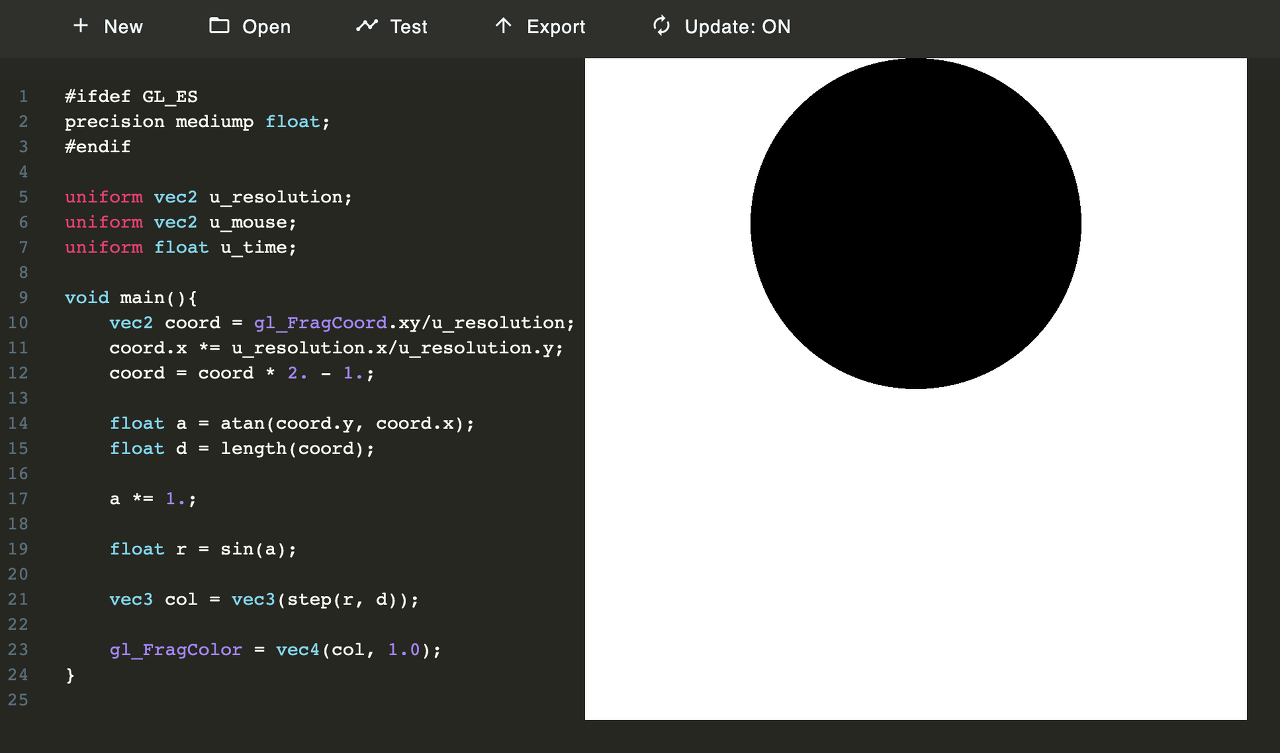
If 'a' is multiplied by 2,
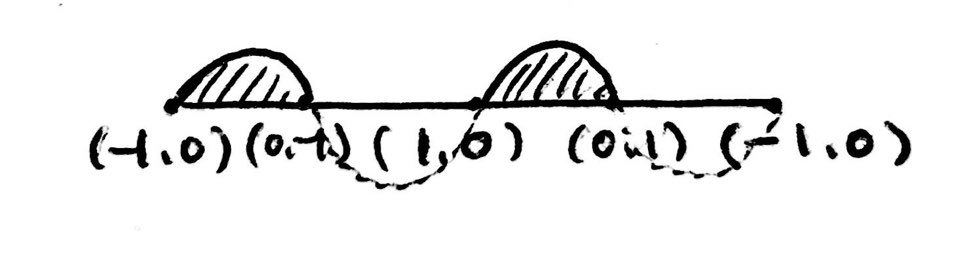
By 3,
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
float a = atan(coord.y, coord.x);
float d = length(coord);
a *= 3.;
float r = sin(a);
vec3 col = vec3(step(r, d));
gl_FragColor = vec4(col, 1.0);
}
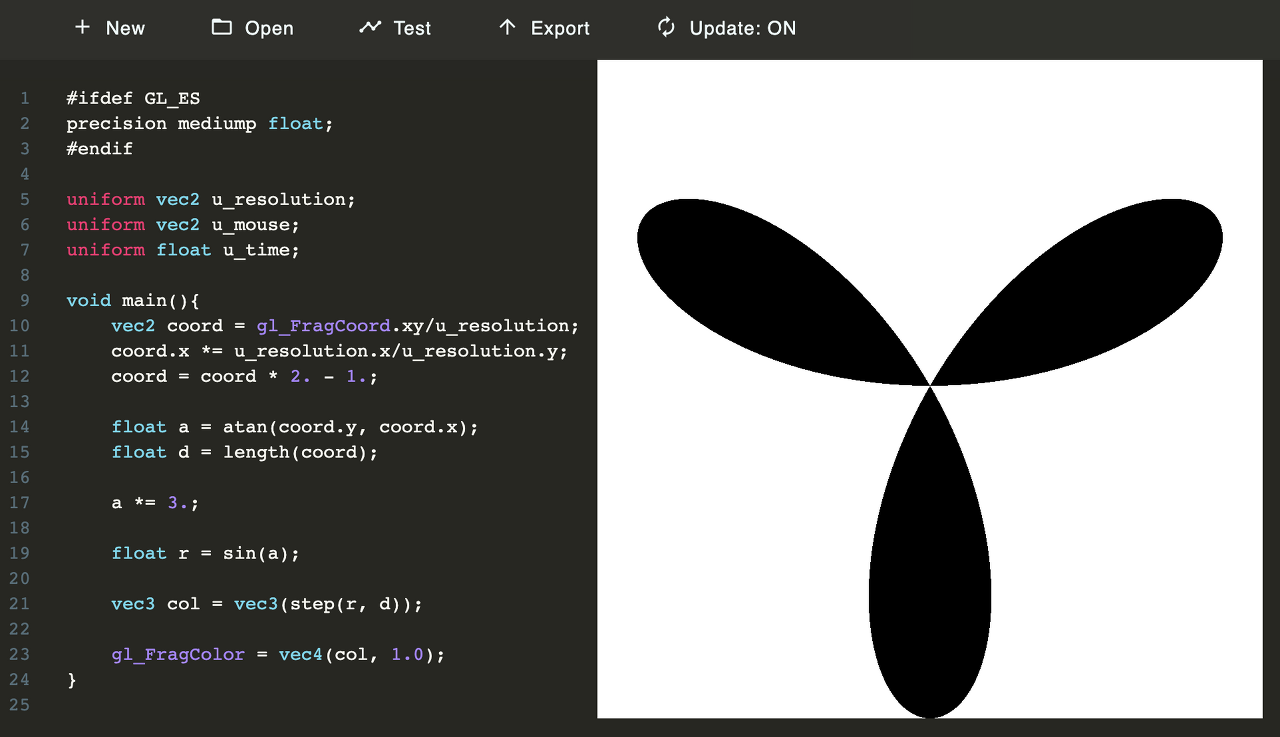
By adding some value to 'a', the sin period can be shifted to output different locations.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
float a = atan(coord.y, coord.x);
float d = length(coord);
a *= 3.;
a += u_time;
float r = sin(a);
vec3 col = vec3(step(r, d));
gl_FragColor = vec4(col, 1.0);
}
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Rotate and Scale (0) | 2022.01.06 |
---|---|
[GLSL] Translate (0) | 2022.01.06 |
[GLSL] Circle Shapes (0) | 2022.01.06 |
[GLSL] Circle (0) | 2022.01.06 |
[GLSL] Rectangle (0) | 2022.01.05 |
댓글