728x90
To make circle, the functions ‘length’ and ‘distance’ are often used.
// Author @patriciogv - 2015
// http://patriciogonzalezvivo.com
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 st = gl_FragCoord.xy/u_resolution;
float pct = 0.0;
vec2 toCenter = vec2(0.5)-st;
pct = length(toCenter);
vec3 color = vec3(pct);
gl_FragColor = vec4( color, 1.0 );
}
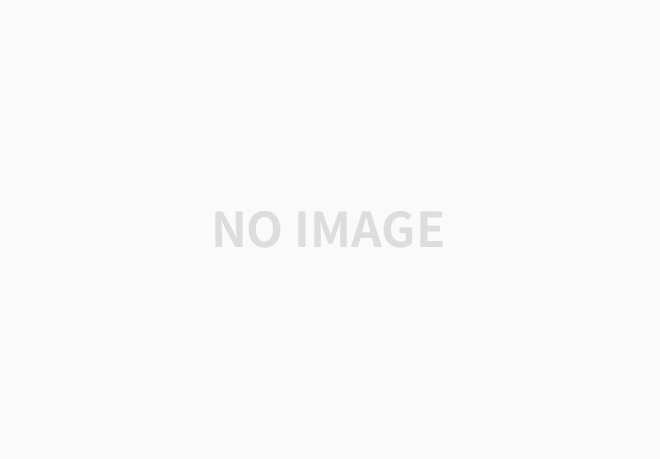
- Use step( ) to turn everything above 0.5 to white and everything below to 0.0.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(step(r, d));
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = circle(coord, vec2(.5), .5);
gl_FragColor = vec4( color, 1.0 );
}
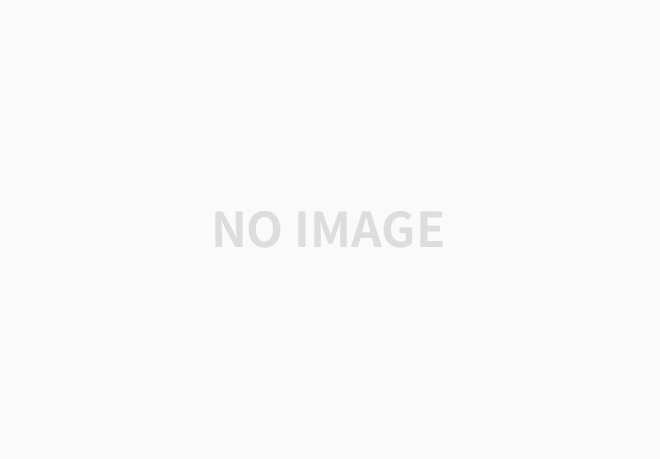
- Inverse the colors of the background and foreground.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(1.-step(r, d));
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = circle(coord, vec2(.5), .5);
gl_FragColor = vec4( color, 1.0 );
}
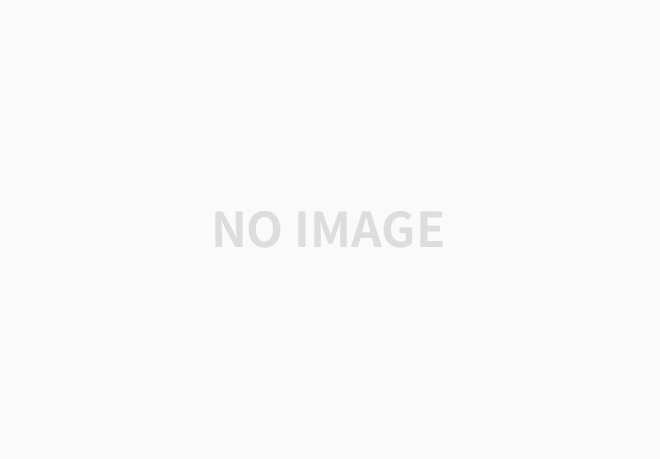
- Using smoothstep( ), experiment with different values to get nice smooth borders on your circle.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(1.-smoothstep(r-0.02, r, d));
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = circle(coord, vec2(.5), .5);
gl_FragColor = vec4( color, 1.0 );
}
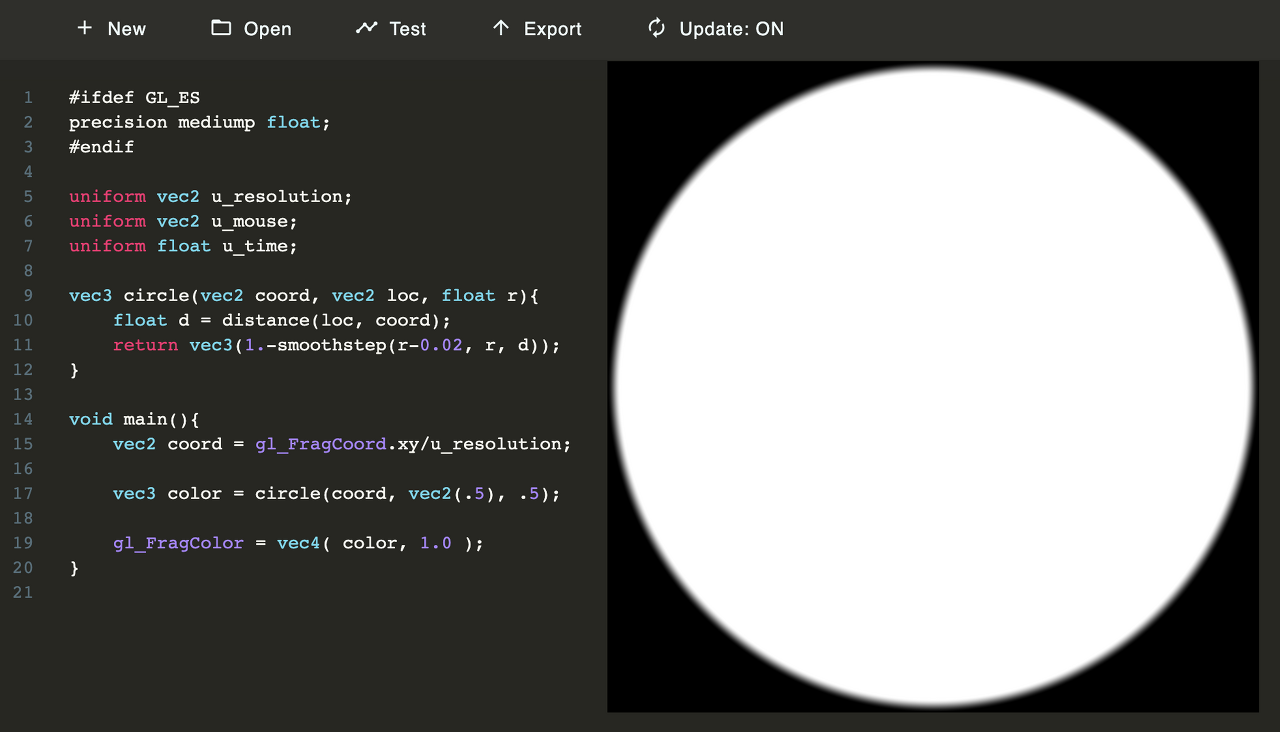
- Add color to the circle.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(1.-smoothstep(r-0.02, r, d), 0, 0);
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = circle(coord, vec2(.5), .5);
gl_FragColor = vec4( color, 1.0 );
}
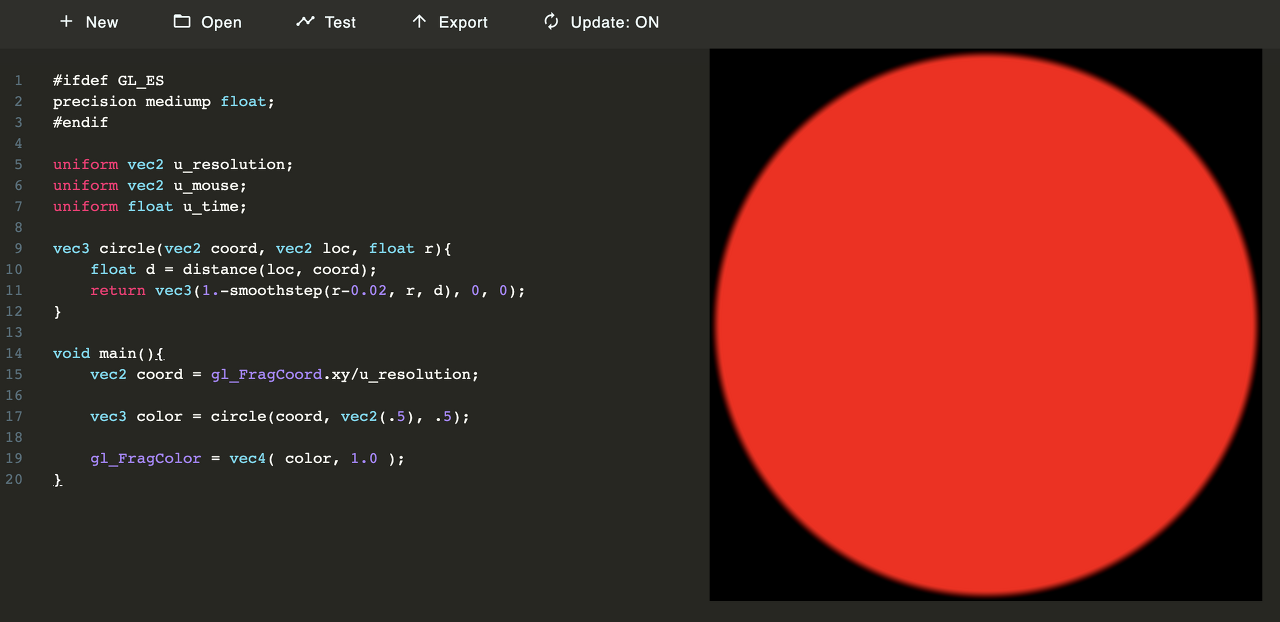
- Can you animate your circle to grow and shrink, simulating a beating heart? (You can get some inspiration from the animation in the previous chapter.)
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(1.-smoothstep(r-0.02, r, d), 0, 0);
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = circle(coord, vec2(.5), abs(sin(u_time*2.))/8.+.375);
gl_FragColor = vec4( color, 1.0 );
}
- What about moving this circle? Can you move it and place different circles in a single billboard?
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
vec3 circle(vec2 coord, vec2 loc, float r){
float d = distance(loc, coord);
return vec3(1.-smoothstep(r-0.02, r, d));
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
vec3 color = vec3(0.);
color = circle(coord, vec2(.25), .25) + circle(coord, vec2(.75), .25);
gl_FragColor = vec4( color, 1.0 );
}
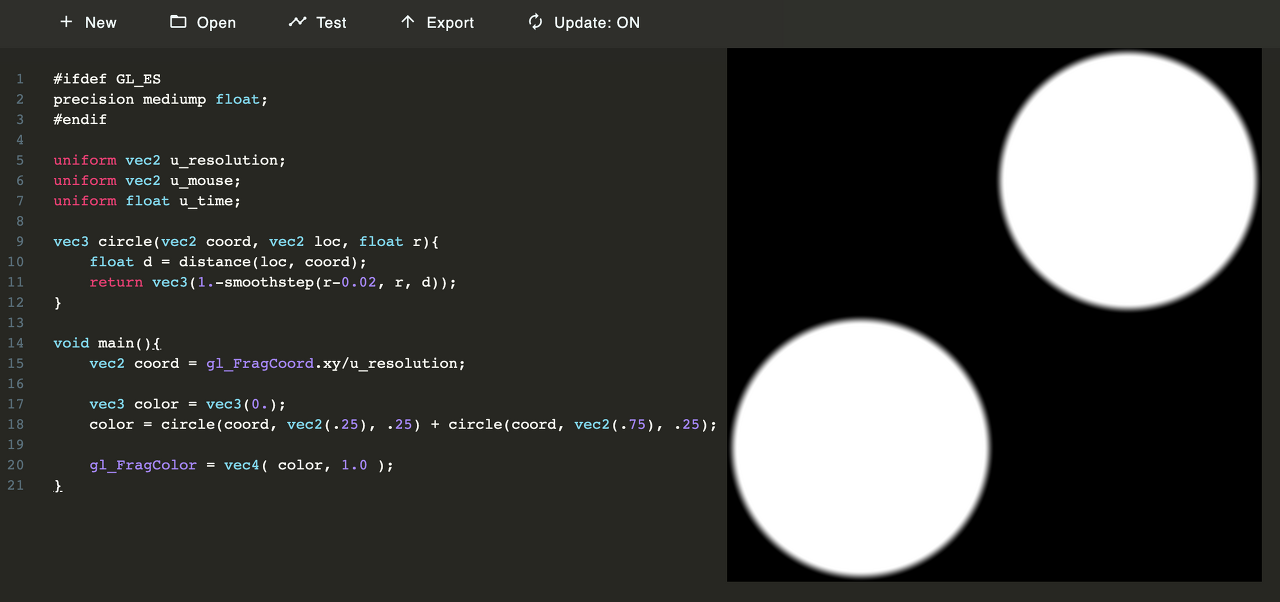
- What happens if you combine distances fields together using different functions and operations?
pct = distance(st,vec2(0.4)) + distance(st,vec2(0.6));
pct = distance(st,vec2(0.4)) * distance(st,vec2(0.6));
pct = min(distance(st,vec2(0.4)),distance(st,vec2(0.6)));
pct = max(distance(st,vec2(0.4)),distance(st,vec2(0.6)));
pct = pow(distance(st,vec2(0.4)),distance(st,vec2(0.6)));
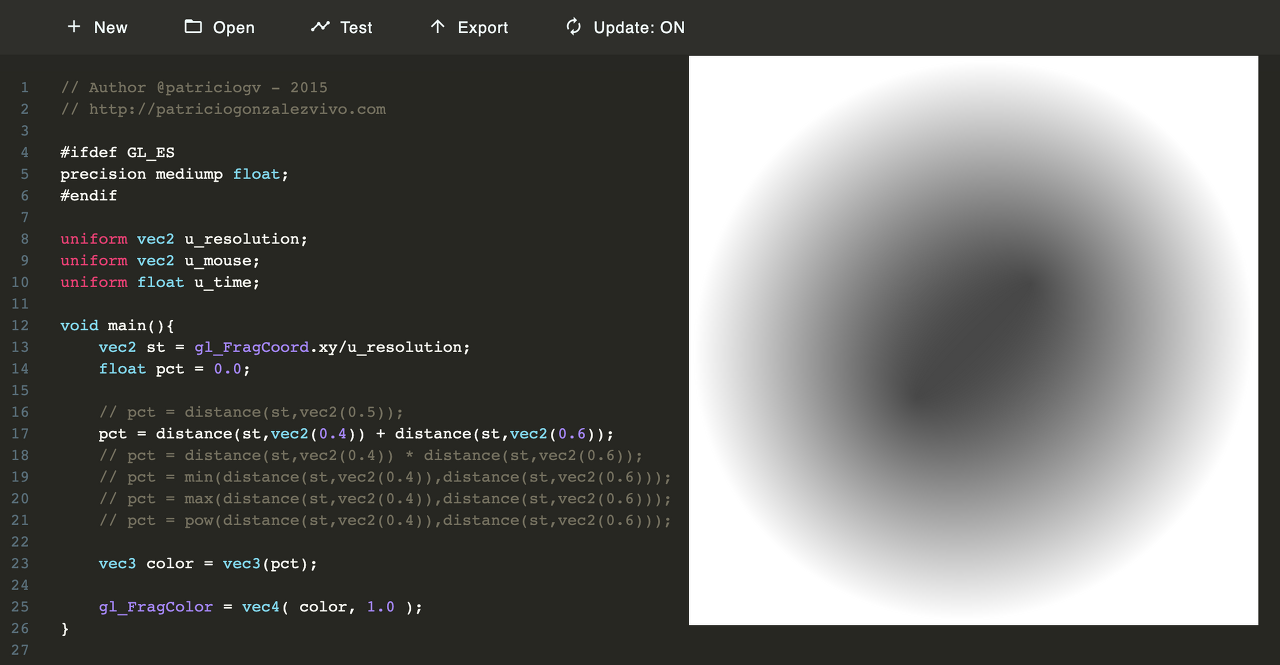
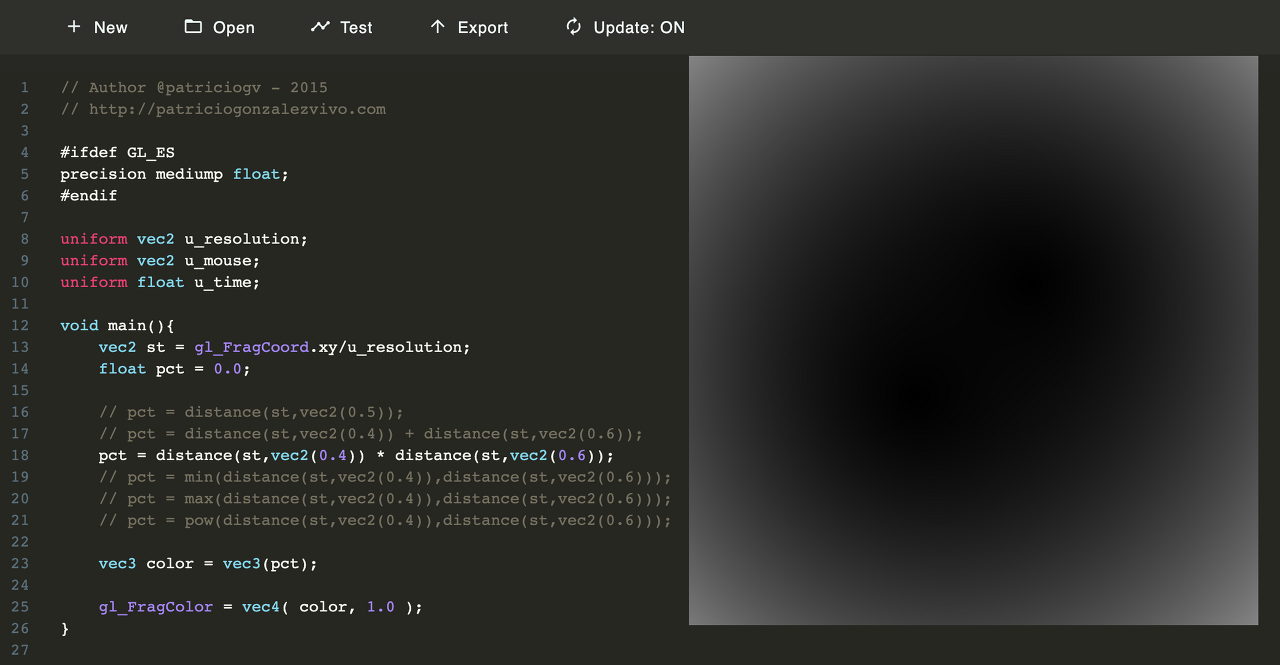
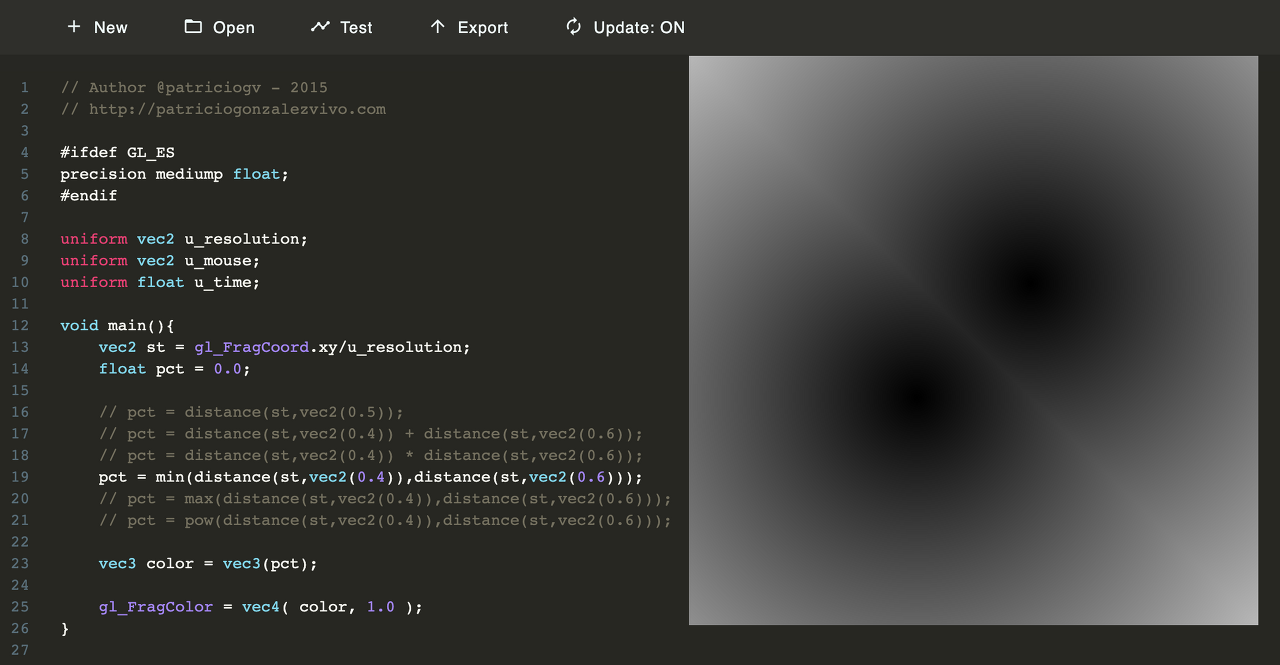
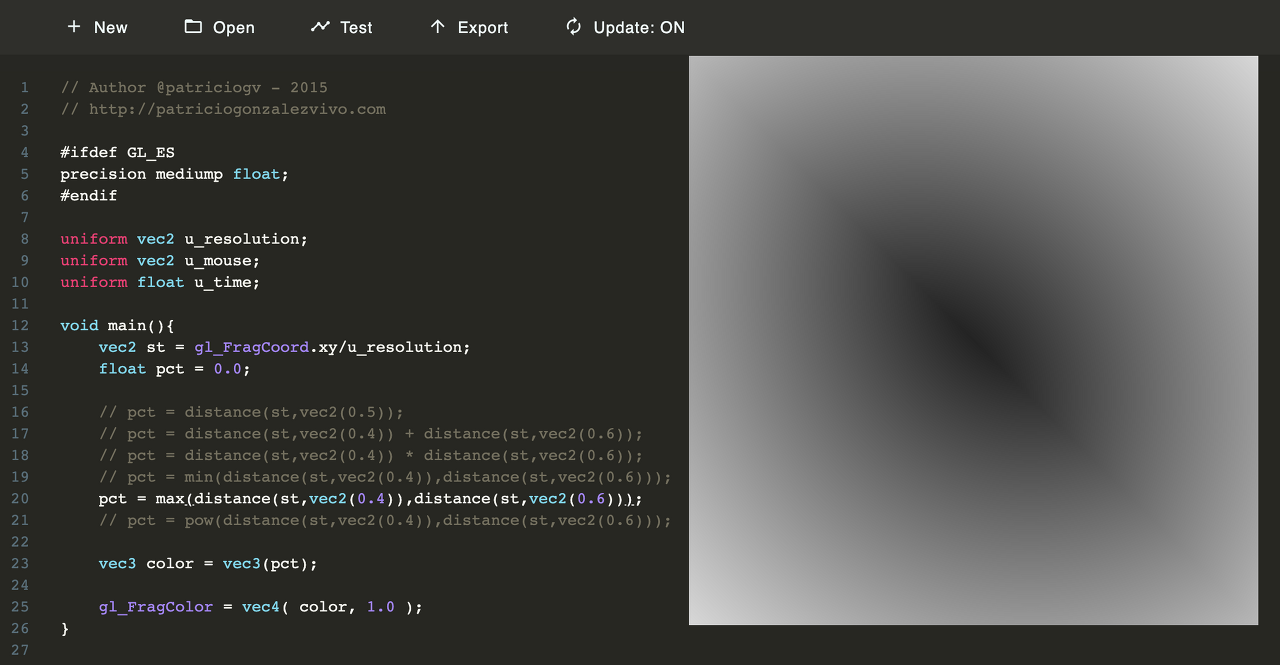
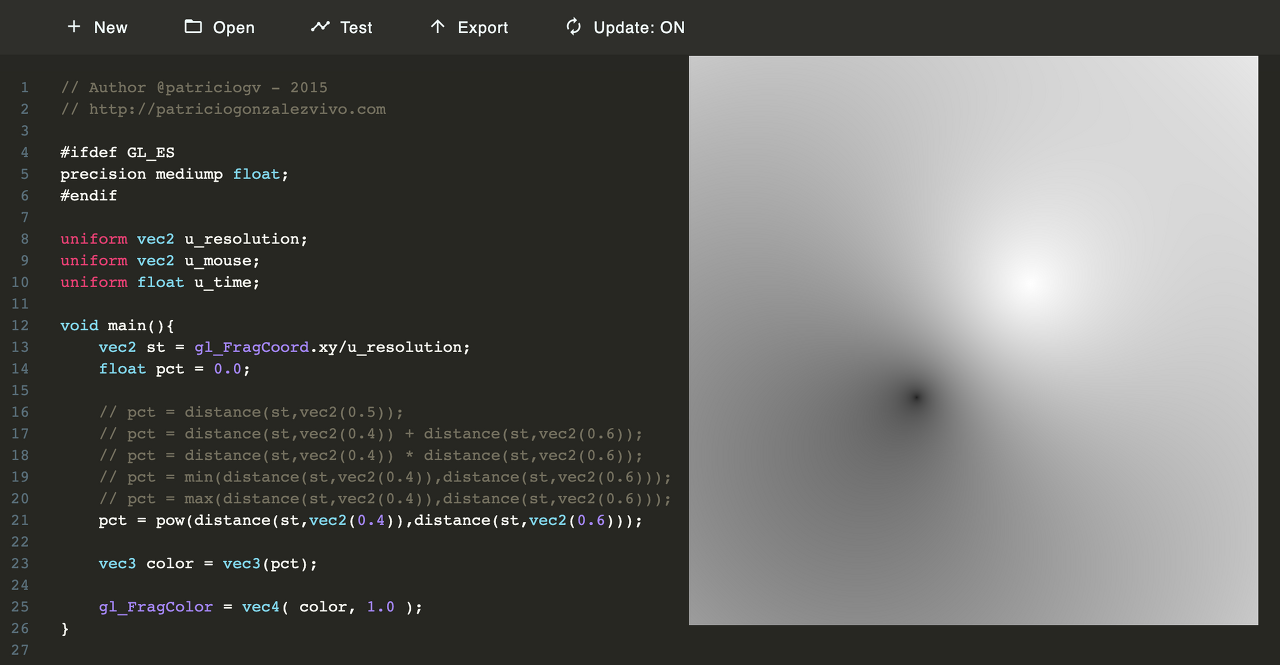
728x90
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Polar Shapes (0) | 2022.01.06 |
---|---|
[GLSL] Circle Shapes (0) | 2022.01.06 |
[GLSL] Rectangle (0) | 2022.01.05 |
[GLSL] Qualifier (0) | 2022.01.05 |
[GLSL] Atan (0) | 2022.01.05 |
댓글