728x90
Let's make mirrored circle wave. New default codes:
#ifdef GL_ES
precision mediump float;
#endif uniform
vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y; // line 11
vec3 col = vec3(.0);
gl_FragColor = vec4(col, 1.0);
}
In the line 11, by multiplying the x-coordinate by the ratio of the horizontal and vertical length of the resolution, the ratio of the picture does not change even if the aspect ratio changes.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
vec2 point = vec2(.3);
float d = distance(coord, point);
vec3 col = vec3(d);
gl_FragColor = vec4(col, 1.0);
}
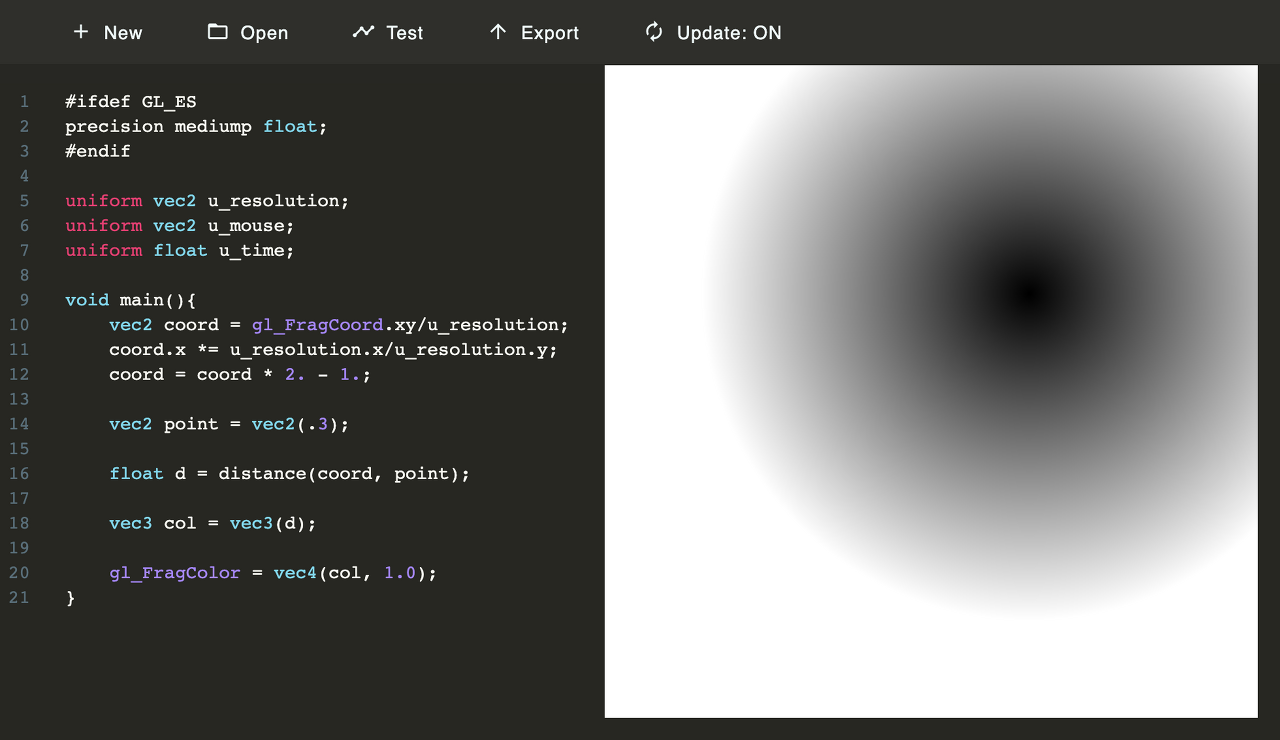
In the line 16 below, change coord to abs(coord) then the points in the second, third and fourth quadrants are also calculated as the distances in the first quadrant. The pixels in the first quadrant appear to have been copied.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
vec2 point = vec2(.3);
float d = distance(abs(coord), point); // line 16
vec3 col = vec3(d);
gl_FragColor = vec4(col, 1.0);
}
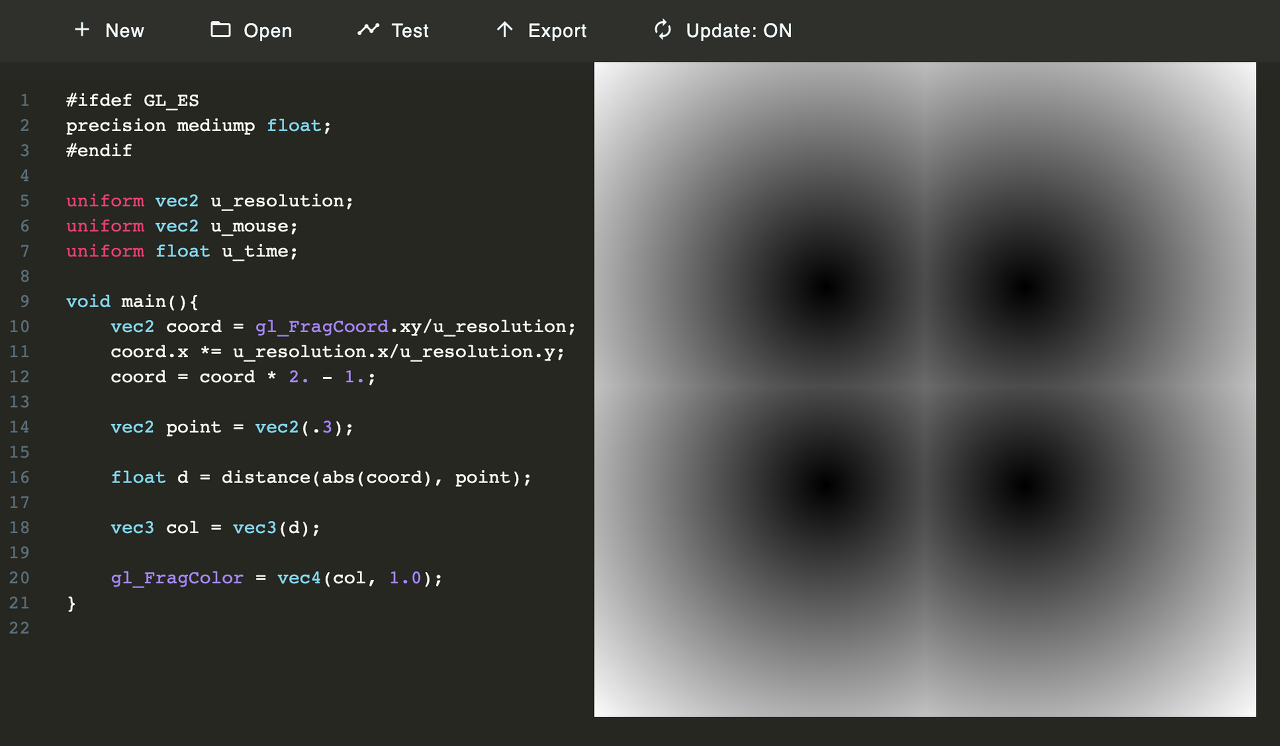
Finally use the function 'fract' to make waves. In the line 18, put 'd' in the function and multiply moderately large number.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord = coord * 2. - 1.;
vec2 point = vec2(.3);
float d = distance(abs(coord), point);
vec3 col = vec3(fract(d*10.)); // line 18
gl_FragColor = vec4(col, 1.0);
}
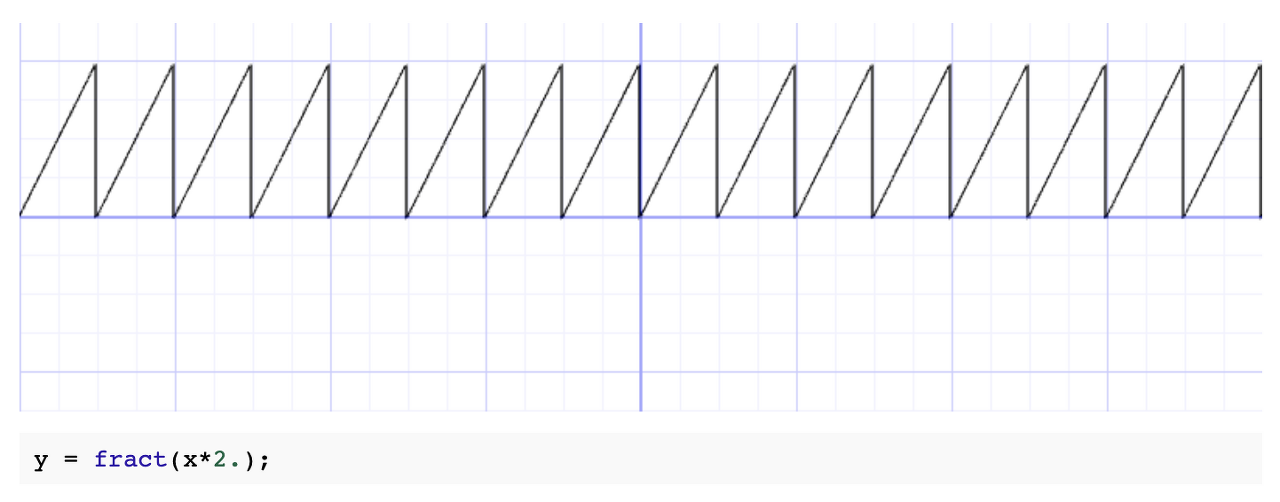
Let's change the aspect ratio:
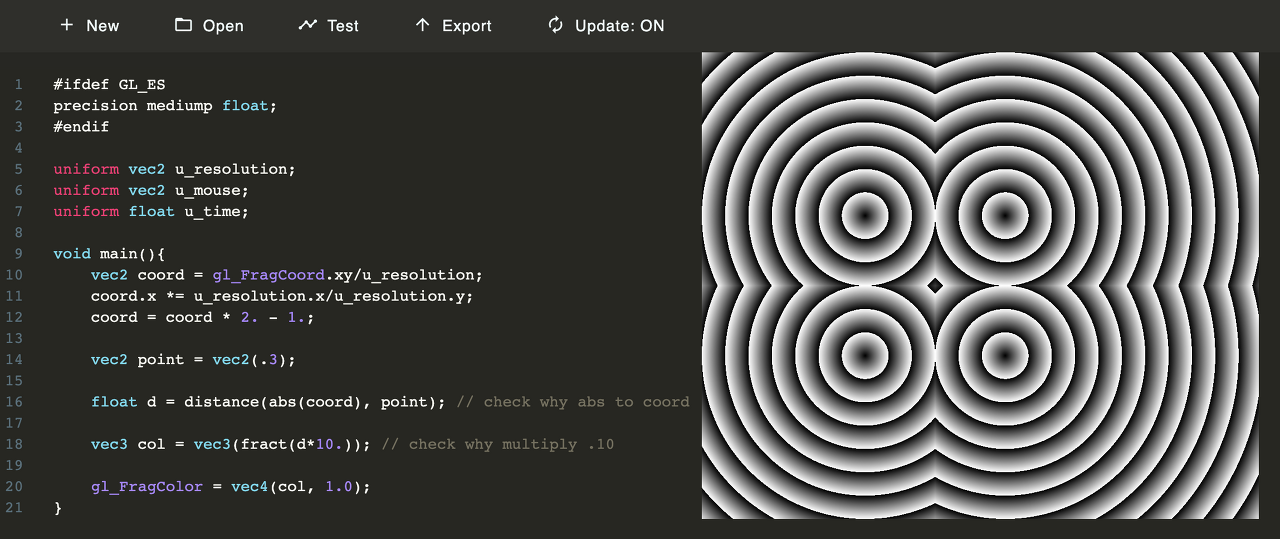
728x90
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Translate (0) | 2022.01.06 |
---|---|
[GLSL] Polar Shapes (0) | 2022.01.06 |
[GLSL] Circle (0) | 2022.01.06 |
[GLSL] Rectangle (0) | 2022.01.05 |
[GLSL] Qualifier (0) | 2022.01.05 |
댓글