728x90
반응형
For the first, let's make a circle.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
float rad = .3;
vec2 center = vec2(.5);
vec3 col = vec3(step(rad, distance(coord, center)));
gl_FragColor = vec4(col, 1.);
}
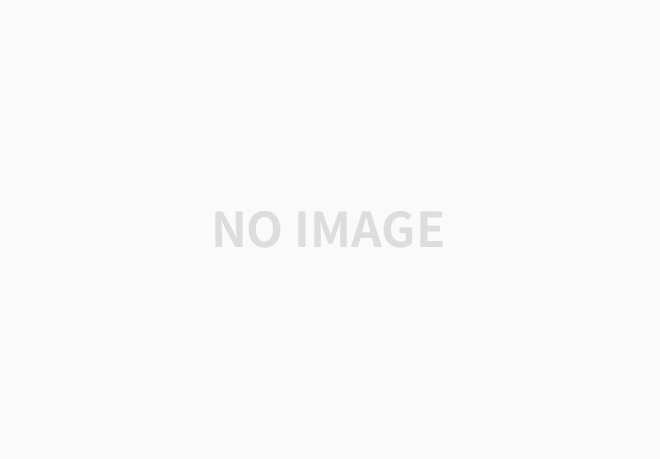
Then, triple the coordinates.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord *= 3.;
float rad = .3;
vec2 center = vec2(.5);
vec3 col = vec3(step(rad, distance(coord, center)));
gl_FragColor = vec4(col, 1.);
}
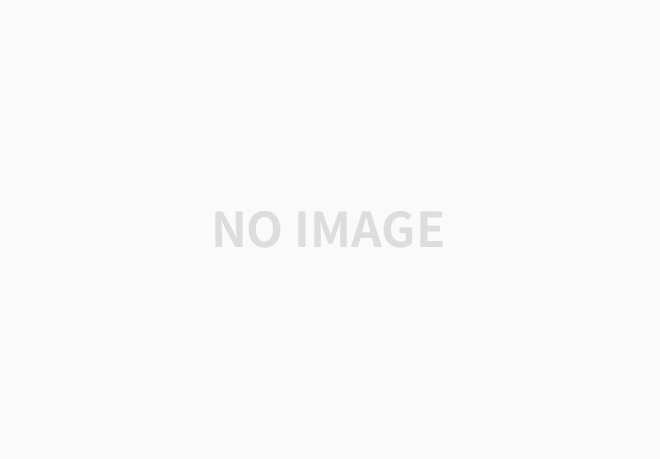
Use the function 'fract',
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord *= 3.;
coord = fract(coord);
float rad = .3;
vec2 center = vec2(.5);
vec3 col = vec3(step(rad, distance(coord, center)));
gl_FragColor = vec4(col, 1.);
}
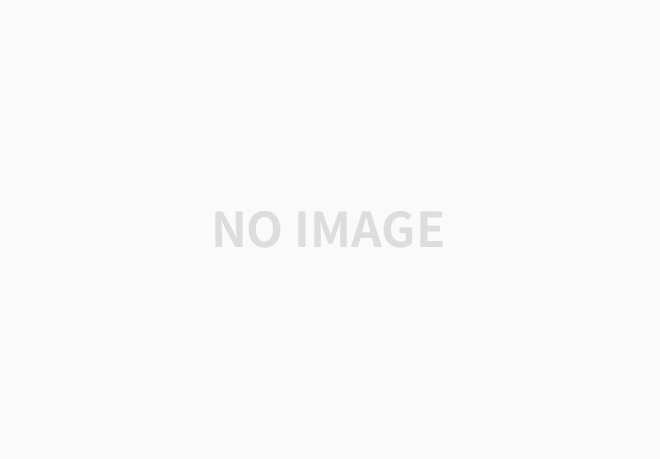
and the coordinates are repeated three times from 0 to 1, from 0 to 1, and from 0 to 1.
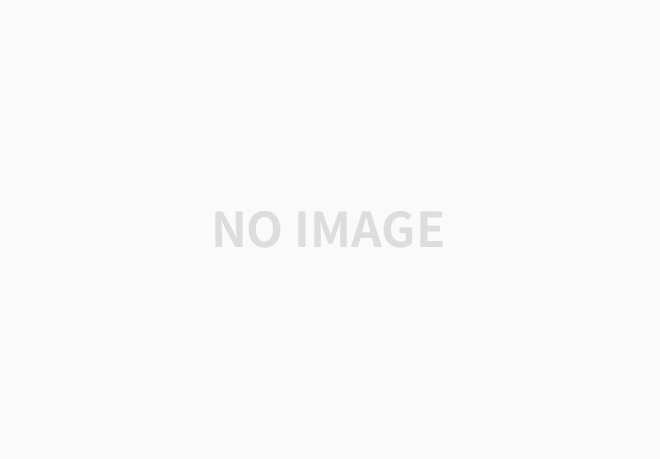
So, we can make a pattern like this.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord *= 3.;
coord = fract(coord);
vec3 col = vec3(coord, 1.);
gl_FragColor = vec4(col, 1.);
}
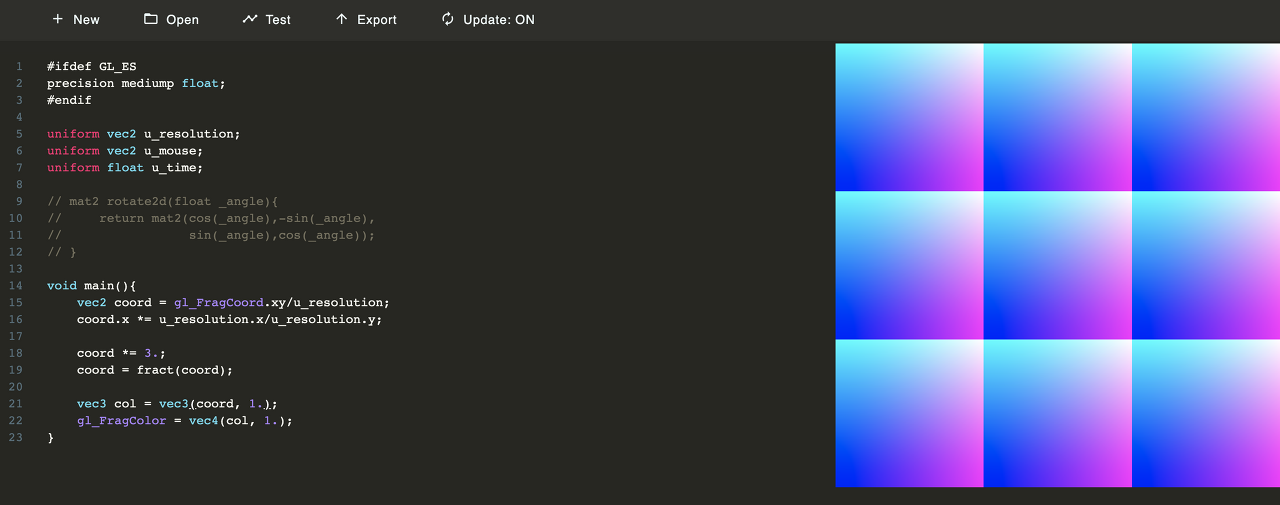
Made another pattern.
#ifdef GL_ES
precision mediump float;
#endif
#define ROOT2 1.414
uniform vec2 u_resolution;
uniform vec2 u_mouse;
uniform float u_time;
mat2 rotate2d(float _angle){
return mat2(cos(_angle),-sin(_angle),
sin(_angle),cos(_angle));
}
float rec(vec2 loc, vec2 size, vec2 coord){
float hor = step(loc.x - size.x, coord.x) - step(loc.x + size.x, coord.x);
float ver = step(loc.y - size.y, coord.y) - step(loc.y + size.y, coord.y);
return hor * ver;
}
void main(){
vec2 coord = gl_FragCoord.xy/u_resolution;
coord.x *= u_resolution.x/u_resolution.y;
coord *= 7.;
coord = fract(coord);
coord -= .5;
coord *= rotate2d(0.785);
vec2 loc = vec2(0.);
vec2 size = vec2(.353);
vec3 col = vec3(rec(loc, size, coord));
gl_FragColor = vec4(col, 1.);
}
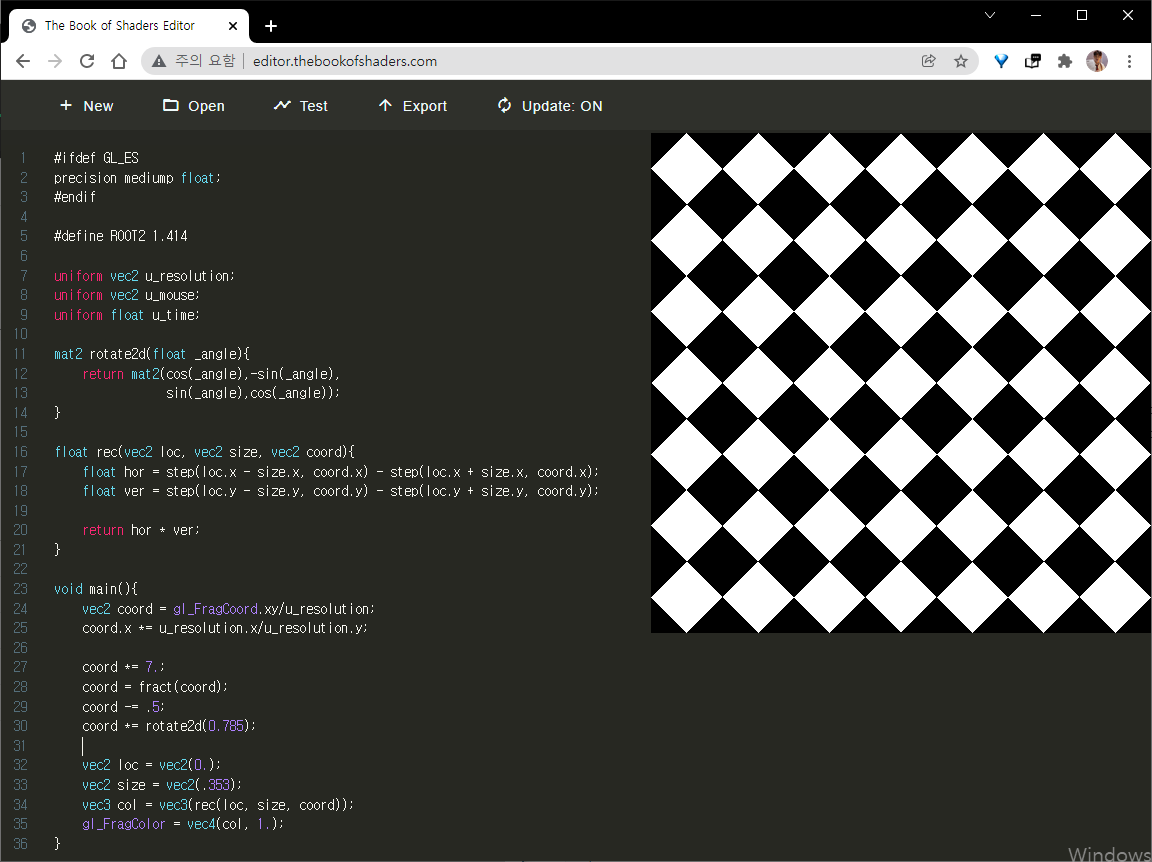
728x90
반응형
'College Study > GLSL' 카테고리의 다른 글
[GLSL] Maze and Glitch (0) | 2022.01.06 |
---|---|
[GLSL] Random (0) | 2022.01.06 |
[GLSL] Rotate and Scale (0) | 2022.01.06 |
[GLSL] Translate (0) | 2022.01.06 |
[GLSL] Polar Shapes (0) | 2022.01.06 |
댓글